To open a browser window inside VS Code you can use the WebView API, though you need to supply HTML content rather than a URL:
export function activate(context: vscode.ExtensionContext) {
context.subscriptions.push(
vscode.commands.registerCommand('catCoding.start', () => {
// Create and show panel
const panel = vscode.window.createWebviewPanel(
'catCoding',
'Cat Coding',
vscode.ViewColumn.One,
{}
);
// And set its HTML content
panel.webview.html = getWebviewContent();
})
);
}
function getWebviewContent() {
return `<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Cat Coding</title>
</head>
<body>
<img src="https://media.giphy.com/media/JIX9t2j0ZTN9S/giphy.gif" width="300" />
</body>
</html>`;
}
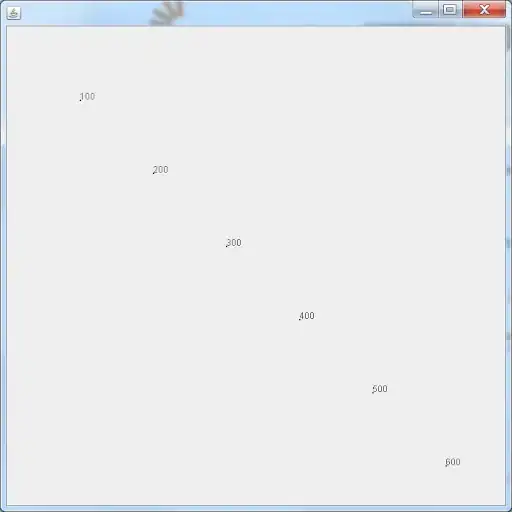
Depending on your specific use case, there's also the Browser Preview extension which registers a command browser-preview.openPreview
that you could use (you'd probably want to list browser-preview as a dependency to ensure it's installed).
And finally, if you just want to open in a normal browser window you can use the env.openExternal
API. When running in remote environments this will also take care of exposing ports and mapping to the exposed hostname (if it's a localhost-served service):
vscode.env.openExternal(Uri.parse("https://www.stackoverflow.com/"));