You should use a UITableView with sections added. There is a pretty good tutorial here.
Updated
First, create a UITableView by dragging on onto your view controller. Add the necessary constraints, and generate a prototype cell.
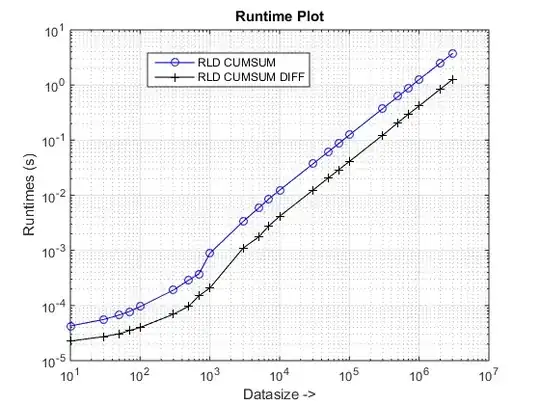
Set the cell reuse identifier as cell.
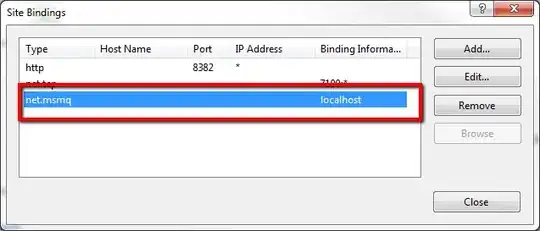
Then, Control Left Click and drag the tableView to the ViewController (the yellow circle on the top). Do this twice and assign it as the DataSource and Delegate.
Open the assistant editor and control drag the tableView to the class to create an IBOutlet. Then add UITableViewDelegate
to your class declaration:
class ViewController: UIViewController, UITableViewDelegate {
Once you have done this, create two new blank Swift files. File, New, File.
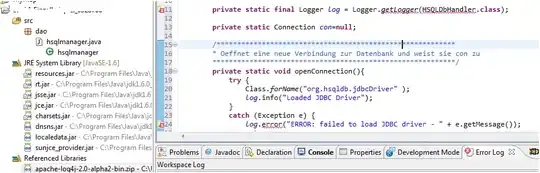
Title one file Section.swift and the other SectionsData.swift.
In the file Section.swift, add this code.
struct Section
{
var heading : String
var items : [String]
init(title: String, objects : [String]) {
heading = title
items = objects
}
}
Here you are defining a structure so the data can be obtained later.
In the SectionsData file put in the following code. This is where you will be able to edit what goes into your table.
class SectionsData {
func getSectionsFromData() -> [Section] {
var sectionsArray = [Section]()
let hello = Section(title: "Hello", objects: ["Create", "This", "To", "The"])
let world = Section(title: "World", objects: ["Extent", "Needed", "To", "Supply", "Your", "Data"])
let swift = Section(title: "Swift", objects: ["Swift", "Swift", "Swift", "Swift"])
sectionsArray.append(hello)
sectionsArray.append(world)
sectionsArray.append(swift)
return sectionsArray
}
}
In this file, you created a class and then a function in order to save and retrieve the data.
Now, in the file with the IBOutlet of your tableview, create the follow variable.
var sections: [Section] = SectionsData().getSectionsFromData()
Now that the hard work is done, time to populate the table. The following functions allow for that.
func numberOfSectionsInTableView(tableView: UITableView) -> Int
{
return sections.count
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
return sections[section].items.count
}
func tableView(tableView: UITableView, titleForHeaderInSection section: Int) -> String?
{
return sections[section].heading
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
{
let cell = tableView.dequeueReusableCellWithIdentifier("cell", forIndexPath: indexPath)
cell.textLabel?.text = sections[indexPath.section].items[indexPath.row]
return cell
}
You should be able to run this and get the results you want. You can edit the cell look when you supply the data. For example,
cell.textLabel?.font = UIFont(name: "Times New Roman", size: 30)
Just make sure when changing the font like that, the string name is exactly how it is spelled.
I hope this helps.