If you want to tackle with watermark attack, I will recommend you image hash algorithms, they are fast and robust to this kind of attack. Let me show you some examples

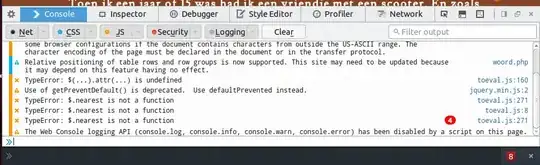
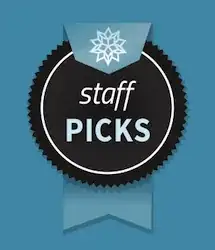
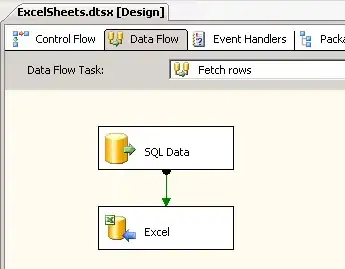
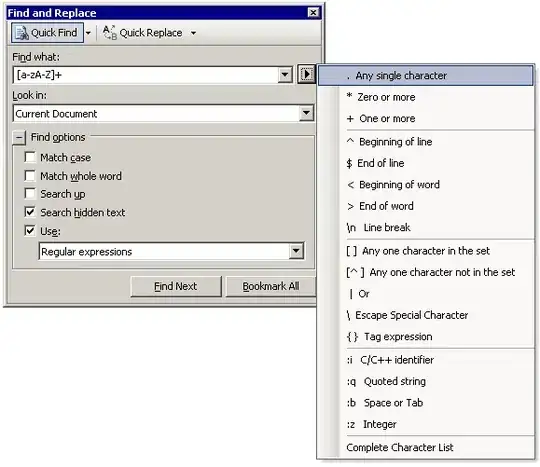
#include <opencv2/core.hpp>
#include <opencv2/core/ocl.hpp>
#include <opencv2/highgui.hpp>
#include <opencv2/img_hash.hpp>
#include <opencv2/imgproc.hpp>
#include <iostream>
void watermark_attack(cv::Ptr<cv::img_hash::ImgHashBase> algo)
{
std::vector<std::string> const origin_img
{
"origin_00.png", "origin_01.png",
"origin_02.png", "origin_03.png"
};
std::vector<std::string> const watermark_img
{
"watermark_00.png", "watermark_01.png",
"watermark_02.png", "watermark_03.png"
};
cv::Mat origin_hash, watermark_hash;
for(size_t i = 0; i != origin_img.size(); ++i){
cv::Mat const input = cv::imread(origin_img[i]);
cv::Mat const watermark_input = cv::imread(watermark_img[i]);
//compute the hash value of image without watermark
algo->compute(input, origin_hash);
//compute the hash value of image with watermark
algo->compute(watermark_input, watermark_hash);
//compare the different between the hash values
//of original image and watermark image
std::cout<<algo->compare(origin_hash, watermark_hash)
<<std::endl;
}
}
int main()
{
using namespace cv::img_hash;
//disable opencl acceleration may(or may not) boost up speed of img_hash
cv::ocl::setUseOpenCL(false);
watermark_attack(AverageHash::create());
}
The results are 1,2,1,2, all pass.
This small program compare the original image(left) with their watermark brother(right), the smaller the value of compute show, more similar the images are. For the case of AverageHash, recommend threshold is 5(that means if the compare result is bigger than 5, the images are consider very different).
Not only watermark, AverageHash provide another "side effects", this algorithm work under contrast , noise(gaussian, pepper and salt), resize and jpeg compression attack too.
The other benefit of using image hash is you can store the hash values of your images in a file, you do not need to compute the hash values again and again.
Simple and fast method to compare images for similarity will show you more details about the img_hash module of opencv.