Details
- swift 4.2
- Xcode 10.1 (10B61)
Solution 1
https://github.com/zixun/CrashEye/blob/master/CrashEye/Classes/CrashEye.swift
Full sample of solution 1
!!! DO NOT FORGET TO COPY (or INSTALL POD) SOLUTION CODE !!!
AppDelegate.swift
import UIKit
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
CrashEye.add(delegate: self)
return true
}
}
extension AppDelegate: CrashEyeDelegate {
func crashEyeDidCatchCrash(with model: CrashModel) {
UserDefaults.standard.set(model.reason + "(\(Date()))", forKey: "crash")
}
}
ViewController.swift
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let button = UIButton(frame: CGRect(x: 40, y: 40, width: 80, height: 44))
button.setTitle("BOOOM", for: .normal)
button.setTitleColor(.blue, for: .normal)
button.addTarget(self, action: #selector(boomButtonTouchedUpInside), for: .touchUpInside)
view.addSubview(button)
}
override func viewDidAppear(_ animated: Bool) {
if let date = UserDefaults.standard.string(forKey: "crash") {
let alert = UIAlertController(title: "", message: date, preferredStyle: .alert)
alert.addAction(UIAlertAction(title: "Cancel", style: .cancel, handler: nil))
present(alert, animated: true)
}
}
@objc func boomButtonTouchedUpInside(_ sender: Any) {
let arr = [1, 2, 3]
let elem = arr[4]
}
}
Solution 2. Crashlytics
Install Crashlytics
Full sample of solution 2
AppDelegate.swift
import UIKit
import Fabric
import Crashlytics
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
Crashlytics.sharedInstance().delegate = self
Fabric.with([Crashlytics.self])
return true
}
}
extension AppDelegate: CrashlyticsDelegate {
func crashlyticsDidDetectReport(forLastExecution report: CLSReport) {
let alert = UIAlertController(title: "\(report.dateCreated)", message: report.identifier, preferredStyle: .alert)
alert.addAction(UIAlertAction(title: "Cancel", style: .cancel, handler: nil))
DispatchQueue.global(qos: .background).async {
sleep(3)
DispatchQueue.main.async {
self.window?.rootViewController?.present(alert, animated: true)
}
}
}
}
ViewController.swift
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let button = UIButton(frame: CGRect(x: 40, y: 40, width: 80, height: 44))
button.setTitle("BOOOM", for: .normal)
button.setTitleColor(.blue, for: .normal)
button.addTarget(self, action: #selector(boomButtonTouchedUpInside), for: .touchUpInside)
view.addSubview(button)
}
@objc func boomButtonTouchedUpInside(_ sender: Any) {
let arr = [1, 2, 3]
let elem = arr[4]
}
}
Results
- Launch app
- Push "Booom" button (app will crash)
Lunch app again
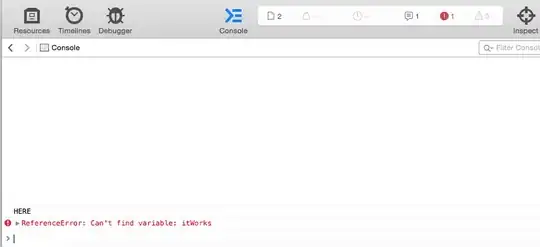