First, what I would do is change the Members
property to be an array
instead of an object
. Then, I would do something like this:
//original json. Notice that I added '[ ]' to make it an array
var json = "{ 'Members': " +
"[ " +
"{'Example1': " +
"{ 'LastOnline': '2016-05-16T12:09:04.6459345Z', " +
"'TimeUntillEx': '2016-05-16T12:09:04.6459345Z', " +
"'Paied': true" +
"}, " +
"} " +
"] " +
"}";
//new member
var newMember = "{ 'Example2': " +
"{ 'LastOnline': '2016-12-16T12:09:04.6459345Z', " +
"'TimeUntillEx': '2016-12-16T12:09:04.6459345Z', " +
"'Paied': false" +
"}, " +
"}";
//parse the json
var obj = JObject.Parse(json);
//get members array
var array = obj.GetValue("Members") as JArray;
//parse the new member and add it to the array
var add = JObject.Parse(newMember);
array.Add(add);
//serialize the json
var output = JsonConvert.SerializeObject(obj, Formatting.Indented);
//print the results
Console.WriteLine(output);
For this, you will need
using Newtonsoft.Json;
using Newtonsoft.Json.Linq;
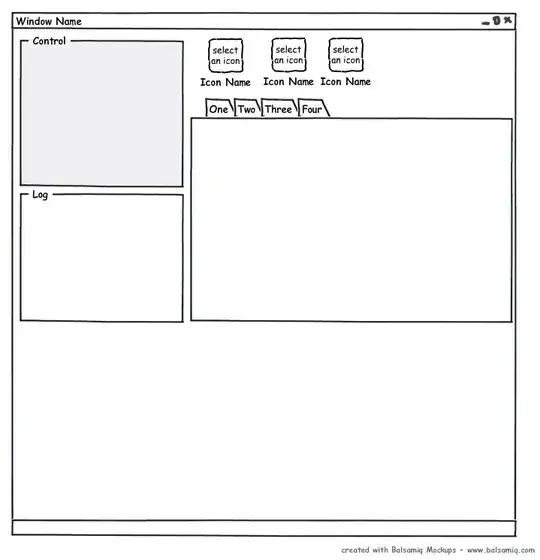