Okay, here is an idea, but I have yet to implement it and check if this actually works.
Mathematically, the two equations describe circles.
Let (a,b) be the center of the first circle and sqrt(r_1) its radius, and let (c,d) be the center of the second circle and sqrt(r_2) its radius. Then, in cartesian coordinates, the points on the circle fullfull respectivelly
(x - a)^2 + (y-b)^2 = r_1
or
(x - c)^2 + (y-d)^2 = r_2
We describe the circle now with two functions: The upper part and the lower part. These are functions involving square roots. So if we have
(x - a)^2 + (y-b)^2 = r_1
Then solving for y gives (via wolfram alpha)
(y-b)^2 = r_1 - (x-a)²
y = b + sqrt(-a²+2ax+r_1-x²)
or
y = b - sqrt(-a²+2ax+r_1-x²)
We can also express the lower and upper part of the other circle with these two equations by exchanging (a,b) with (c,d) and r_1 with r_2.
The point is, once we have two graphs with y_1 = f(x) and y_2 = g(x), then we can find their intersection with f(x) = g(x) or equivalently f(x) - g(x) = 0. For this, we can use approximate solutions found by the Newton's iteration method! We can also compute the needed derivatives:
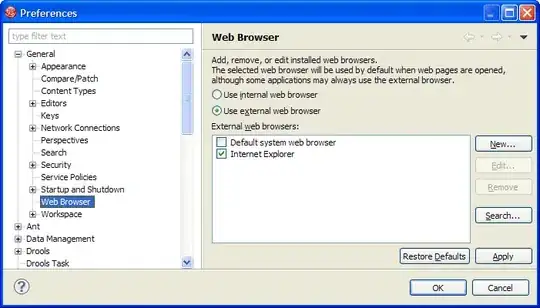
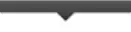
So, the whole idea is that we split each circle in two functions: Upper andl lower part. Then, we check if the upper part of the first circle intersects the function describing the upper part of the second circle or the lower part of the second circle. Same with the lower part, we check it agains the upper and lower part of the other function. And for finding the intersection, we can use the approximate Newton method.
So, for the above example:
(x-0)^2+(y-5)^2=12,25
We get the upper and lower functions as
y = 5 + sqrt(12.25-x^2)
y = 5 - sqrt(12.25 -x^2)
And we can plot them nicely
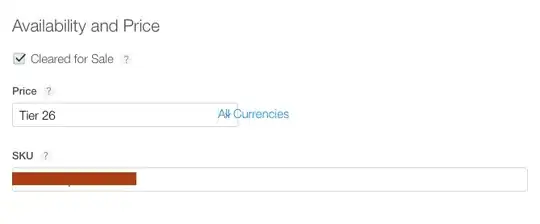
Conversely, the second circle ((x-0)^2+(y-0)^2=2,25
) is described by the equations
y = 0 + sqrt(2.25-x^2)
y = 0 - sqrt(2.25-x^2)
Now, if we look at all these graphs at once:
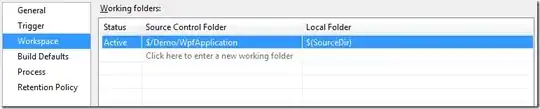
We find that there is an intersection! :). Between the lower part of the first circle and the upper part of the second circle. If we now form the difference between these two functions, we get the following graph for the functions:
f(x) = 5 - sqrt(12.25 -x^2)
g(x) = 0 + sqrt(2.25-x^2)
f(x)-g(x) = 5 - sqrt(12.25 -x^2) - sqrt(2.25-x^2)
And if we plot that
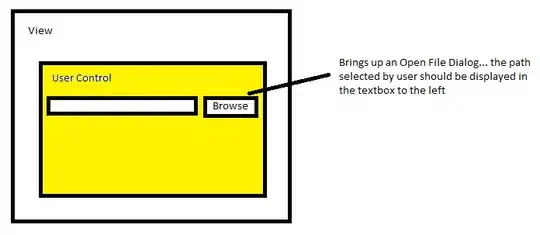
We can see that if we find the zeroes of that graph, we will get the correct solution x = 0! :)
Once we have that solution, we can eliminate one variable in either of the equations
(x-a)^2 + (y-b)^2 = r_1
And we will receive an equation ONLY quadratic in y, which can be solved by the general solution formula (pq-formula or abc-formula).
Hope this gives you some ideas.