If I am not mistaken, you just need to rotate the image. You can do this by applying a layout transform to Image
element in XAML and changing it's (transform's) angle value on button click. Also it looks like you are not following MVVM. If you do, see how simple it is:
View
<Image Source="C:\Users\Public\Pictures\Sample Pictures\Penguins.jpg"
HorizontalAlignment="Center" VerticalAlignment="Center" Width="125">
<Image.LayoutTransform>
<RotateTransform Angle="{Binding RotateAngle}" />
</Image.LayoutTransform>
</Image>
<Button Content="Rotate" Command="{Binding RotateCommand}"
VerticalAlignment="Bottom" HorizontalAlignment="Center" />
ViewModel
public class ViewModel : BaseViewModel
{
private ICommand rotateCommand;
private double rotateAngle;
public ICommand RotateCommand
{
get
{
if(rotateCommand == null)
{
rotateCommand = new RelayCommand(() => {
RotateAngle += 90;
});
}
return rotateCommand;
}
}
public double RotateAngle
{
get
{
return rotateAngle;
}
private set
{
if(value != rotateAngle)
{
rotateAngle = value;
OnPropertyChanged("RotateAngle");
}
}
}
}
View Code-behind
ViewModel vm;
public View()
{
InitializeComponent();
vm = new ViewModel();
DataContext = vm;
}
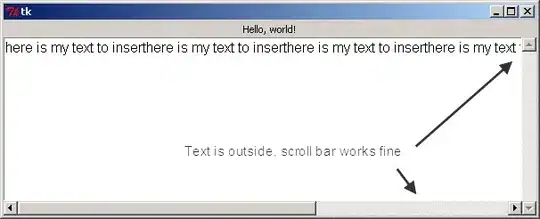
I am assuming you are not absolute beginner in MVVM/WPF and omitted definitions of BaseViewModel (implements INotifyPropertyChanged) and RelayCommand (implements ICommand) as I didn't want to make answer too lengthy. If you are having trouble with these, let me know, I will include them here.