The problem is that your pseudo-element is consuming all available space on the last row.
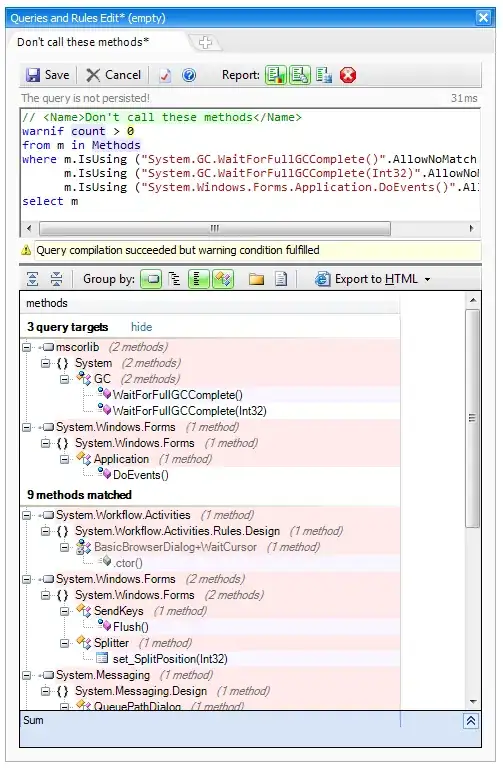
Yes, this is done to neutralize the effect of justify-content: space-between
on two flex items, which causes them to appear on opposite ends...
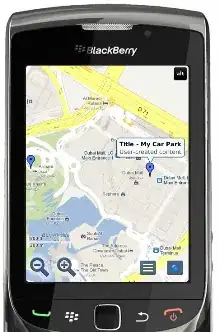
... but it also kills the proportional spacing that space-between
provides when the items fill the row.
Until the flex specification is revised with specific last-row alignment properties, you could implement a hack that solves this problem:
Add invisible flex items after the last real item.
In the example below, I've added seven phantom items to your code.
.container {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
}
/*
.container:after {
flex: 1;
content: '';
}
*/
.invisible { visibility: hidden; }
.container form {
width: 100%;
display: flex;
}
.container .comment {
flex: 1;
}
.square {
padding: 10px;
width: calc(100% / 9);
margin: 0.7vw 0 0.7% 1vw;
background: red;
}
<div class="container">
<form method="post" style="margin: 0.7vw 0 0.7% 1vw;">
<input class="comment" type="text">
<input type="submit">
</form>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square"></div>
<div class="square invisible"></div>
<div class="square invisible"></div>
<div class="square invisible"></div>
<div class="square invisible"></div>
<div class="square invisible"></div>
<div class="square invisible"></div>
<div class="square invisible"></div>
</div>
Revised Fiddle
More information:
Sidenote
There's a good chance you'll encounter some trouble with this rule:
.square { margin: 0.7vw 0 0.7% 1vw; }
The flexbox specification advises against using percentage margin and padding in a flex container.
Authors should avoid using percentages in paddings or margins on flex items entirely, as they will get different behavior in different browsers.
Here's some more:
4.2. Flex Item Margins and Paddings
Percentage margins and paddings on flex items can be resolved against either:
- their own axis (left/right percentages resolve against width, top/bottom resolve against height), or,
- the inline axis (left/right/top/bottom percentages all resolve against width)
A User Agent must choose one of these two behaviors.
Note: This variance sucks, but it accurately captures the current state of the world (no consensus among implementations, and no consensus within the CSSWG). It is the CSSWG’s intention that browsers will converge on one of the behaviors, at which time the spec will be amended.