This type of functionality should ideally be done on the client(in javascript).Here's a complete working example, just copy and paste as is into your solution and it will work:
Code behind:
public partial class GridTwo : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
List<OrderLineItem> lineItems = new List<OrderLineItem>();
lineItems.Add(new OrderLineItem { Price = 10.00M, Quantity = 0, ItemTotal = 0.00M });
lineItems.Add(new OrderLineItem { Price = 100.00M, Quantity = 0, ItemTotal = 0.00M });
lineItems.Add(new OrderLineItem { Price = 5.00M, Quantity = 0, ItemTotal = 0.00M });
GridView1.DataSource = lineItems;
GridView1.DataBind();
}
}
public class OrderLineItem
{
public decimal Price { get; set; }
public int Quantity { get; set; }
public decimal ItemTotal { get; set; }
}
.ASPX:
<head runat="server">
<title></title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
$(".QuantityClass").on('change keyup paste', function () {
//Ctrl+Shift+J in Google Chrome to bring up the console which allows you to debug javascript
debugger;
var textBox = this;
var quantity = $(textBox).val();
var tableRows = $(textBox).parent().parent().children();
if (quantity != "") {
var price = tableRows[0].children[0].innerHTML;
var itemTotal = price * quantity;
tableRows[2].children[0].innerHTML = itemTotal;
}
else
tableRows[2].children[0].innerHTML = "";
});
});
</script>
</head>
<body>
<form id="form1" runat="server">
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:TemplateField HeaderText="Price">
<ItemTemplate>
<asp:Label ID="lblPrice" runat="server" Text='<%# Bind("Price")%>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Quantity">
<ItemTemplate>
<asp:TextBox ID="txtQuantity" CssClass="QuantityClass" runat="server" Text='<%# Bind("Quantity")%>'></asp:TextBox>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Item Total">
<ItemTemplate>
<asp:Label ID="lblItemTotal" runat="server"></asp:Label>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
</form>
</body>
Output:
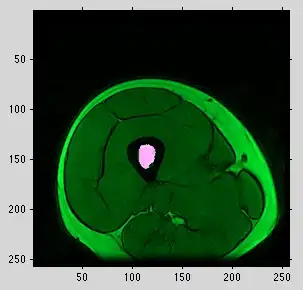