Here's a Sweave file that will conditionally color xtable cells red or green. The conditional formatting of each cell is accomplished with the LaTeX colortbl and xcolor packages.
Coloring xtable cells with Sweave involves pasting together an escape character ("\"), the LaTeX "\cellcolor" function, an HTML argument, your color choice, and your cell value. You'll want to have columns that look something like this before you convert the data.frame to xtable:
c("\\cellcolor[HTML]{FF0600}{1}",
"\\cellcolor[HTML]{2DB200}{}",
"\\cellcolor[HTML]{2DB200}{}")
Here is the full Sweave file. I am compiling this in RStudio using knitr and pdfLaTeX, and I'm previewing it in Sumatra.
\documentclass{article}
\usepackage[table]{xcolor}
\begin{document}
<<xtable1, results = 'asis', echo = FALSE, warning = FALSE>>=
library(xtable)
# build your data.frame
id <- c("1_1", "1_2", "2_1")
a <- c("1", "", "")
b <- c("", "1", "")
c <- c("", "", "1")
d <- data.frame(id, a, b, c)
# define function that will color blank cells green and not blank cells red
color_cells <- function(df, var){
out <- ifelse(df[, var]=="",
paste0("\\cellcolor[HTML]{2DB200}{", df[, var], "}"),
paste0("\\cellcolor[HTML]{FF0600}{", df[, var], "}"))
}
# apply coloring function to each column you want
d$a <- color_cells(df = d, var= "a")
d$b <- color_cells(df = d, var= "b")
d$c <- color_cells(df = d, var= "c")
# convert data.frame to xtable and print it with sanitization
dx <- xtable(d)
align(dx) <- "|c|c|c|c|c|"
print(dx,
hline.after=-1:3,
sanitize.text.function = function(x) x)
@
\end{document}
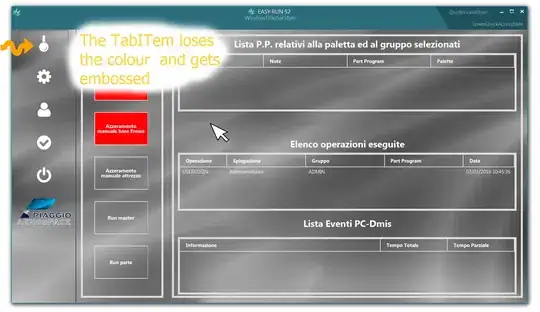
Edit
Here is the code for compiling with Sweave, not knitr:
\documentclass{article}
\usepackage[table]{xcolor}
\begin{document}
\SweaveOpts{concordance=TRUE}
<<xtable1, results = tex, echo = FALSE, warning = FALSE>>=
library(xtable)
# build your data.frame
id <- c("1_1", "1_2", "2_1")
a <- c("1", "", "")
b <- c("", "1", "")
c <- c("", "", "1")
d <- data.frame(id, a, b, c)
# define function that will color NA cells green and not-NA cells red
color_cells <- function(df, var){
out <- ifelse(df[, var]=="",
paste0("\\cellcolor[HTML]{2DB200}{", df[, var], "}"),
paste0("\\cellcolor[HTML]{FF0600}{", df[, var], "}"))
}
# apply coloring function to each column you want
d$a <- color_cells(df = d, var= "a")
d$b <- color_cells(df = d, var= "b")
d$c <- color_cells(df = d, var= "c")
# convert data.frame to xtable and print it with sanitization
dx <- xtable(d)
align(dx) <- "|c|c|c|c|c|"
print(dx,
hline.after=-1:3,
sanitize.text.function = function(x) x)
@
\end{document}