In the example below, each panel in the tabbed pane has its own JComboBox
that listens to a common ComboBoxModel
. When the common model is updated, by clicking Update, each listening JComboBox
sees the change.
I never need any two combo boxes to share a model. My panels contain completely different JComponent
instances, but the look and data those components display depend on several collections common to all of those panels.
You may be able to leverage the observer pattern, examined here. The exact details depend on your use case, but the PropertyChangeListener
examples are worth studying.
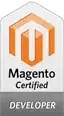
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.util.Date;
import javax.swing.AbstractAction;
import javax.swing.DefaultComboBoxModel;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTabbedPane;
/**
* @see https://stackoverflow.com/a/37514928/230513
* @see https://stackoverflow.com/questions/8752037
* @see https://stackoverflow.com/a/37222598/230513
*/
public class TabTest {
private static final int N = 5;
private final JTabbedPane pane = new JTabbedPane();
private final DefaultComboBoxModel model = new DefaultComboBoxModel(
new String[]{"Alpher", "Bethe", "Gamow", "Dirac", "Einstein"});
public TabTest() {
for (int i = 0; i < N; i++) {
Color color = Color.getHSBColor((float) i / N, 1, 1);
pane.add("Tab " + String.valueOf(i), new TabContent(i, color));
}
}
private class TabContent extends JPanel {
private TabContent(int i, Color color) {
setOpaque(true);
setBackground(color);
add(new JComboBox(model));
}
@Override
public Dimension getPreferredSize() {
return new Dimension(320, 240);
}
}
private void display() {
JFrame f = new JFrame("TabColors");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.add(pane);
JPanel p = new JPanel(new FlowLayout(FlowLayout.RIGHT));
p.add(new JButton(new AbstractAction("Update") {
@Override
public void actionPerformed(ActionEvent e) {
model.addElement(new Date());
}
}));
f.add(p, BorderLayout.SOUTH);
f.pack();
f.setLocationRelativeTo(null);
f.setVisible(true);
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
new TabTest().display();
}
});
}
}