Keeping Your Animals Contained
Consider creating a container for all of your game elements as you only want to randomize their order as you don't want to get the title mixed up in all of this :
<div class='game-container'>
<div class="penguin1"></div>
<div class="penguin2"></div>
<div class="penguin3"></div>
<div class="penguin4"></div>
<div class="penguin5"></div>
<div class="penguin6"></div>
<div class="penguin7"></div>
<div class="penguin8"></div>
<div class="yeti">
</div>
Shuffling Them Around
This should make them easier to randomize through a simple jQuery extension function like this one mentioned in this related thread :
$.fn.randomize = function(selector){
(selector ? this.find(selector) : this).parent().each(function(){
$(this).children(selector).sort(function(){
return Math.random() - 0.5;
}).detach().appendTo(this);
});
return this;
};
You can combine these two approaches by then simply calling the following when your page has loaded :
$(document).ready(function(){
// Define your randomize function here
// Randomize all of the elements in your container
$('.game-container').randomize('div');
});
Example
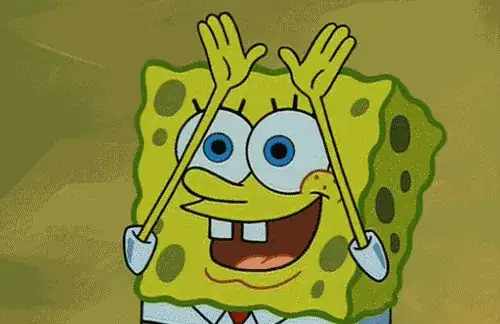
$.fn.randomize = function(selector){
(selector ? this.find(selector) : this).parent().each(function(){
$(this).children(selector).sort(function(){
return Math.random() - 0.5;
}).detach().appendTo(this);
});
return this;
};
// Randomize all of the <div> elements in your container
$(".game-container").randomize('div');
.game-container { width: 300px; }
.penguin { background: url('http://vignette1.wikia.nocookie.net/farmville/images/b/be/Baby_Penguin-icon.png/revision/latest?cb=20110103080900'); height: 100px; width: 100px; display: inline-block; }
.yeti { background: url('http://www.badeggsonline.com/beo2-forum/images/avatars/Yeti.png?dateline=1401638613'); height: 100px; width: 100px; display: inline-block; }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.0/jquery.min.js"></script>
<div class='game-container'>
<div class='penguin'></div>
<div class='penguin'></div>
<div class='penguin'></div>
<div class='penguin'></div>
<div class='penguin'></div>
<div class='penguin'></div>
<div class='penguin'></div>
<div class='yeti'></div>
<div class='penguin'></div>
</div>