Typically you will want to avoid appending data to an array within a loop like you have written it as this has to re-allocate memory every time through the loop as you expand the variable.
The easiest thing to do would be to convert them both to row vectors using (:).'
, concatenate them along the first dimension, and then flatten to a column vector using reshape
. Because of MATLAB's column-major ordering, this will automatically inter-leave the values of A
and B
to create C
.
C = reshape(cat(1, A(:).', B(:).'), [], 1)
Benchmark
As far as whether this is faster than indexing (@ThP's answer), here is a brief test to benchmark the two.
sizes = round(linspace(100, 10000, 100));
times1 = zeros(size(sizes));
times2 = zeros(size(sizes));
for k = 1:numel(sizes)
A = rand(sizes(k), 1);
B = rand(sizes(k), 1);
times1(k) = timeit(@()combine1(A,B));
times2(k) = timeit(@()combine2(A,B));
end
figure
plot(sizes, times1)
hold on
plot(sizes, times2)
legend('cat + reshape', 'Indexing')
function C = combine1(A, B)
C = reshape(cat(1, A(:).', B(:).'), [], 1);
end
function C = combine2(A,B)
C = zeros(2*numel(A),1);
C(1:2:end) = A;
C(2:2:end) = B;
end
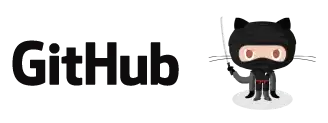