If you want to use a "master" header...
If you want to use a "master" header that would apply this to all of your child headers, then you could use a similar approach to your original example and target the mouseover
and mouseleave
events for your main header and then apply the animation to all of the child elements :
<div id='main-header' class="row">
<div class="col-md-4 header" ><img src="http://www.mandroid.in/demo/srems/images/news_image_01.jpg" /></div>
<!-- More headers omitted for brevity -->
</div>
along with :
// When your main header is hovered, animate all of the sub headers
$("#main-header").mouseleave(function () {
$('.header').animate({ height: "50px" }, 600);
});
$("#main-header").mouseenter(function () {
$('.header').animate({ height: "100px" }, 600);
});
Example (Single Main Header)
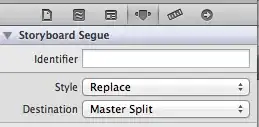
$("#main-header").mouseleave(function() {
$('.header').animate({
height: "50px"
}, 600);
});
$("#main-header").mouseenter(function() {
$('.header').animate({
height: "100px"
}, 600);
});
body {
height: 1000px;
width: 100%;
background-color: #F0F0F0;
}
#header_div {
width: 100%;
height: 100px;
position: fixed;
top: 0;
left: 0;
}
#header_div img {
max-height: 100%;
max-width: 100%;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div id='main-header' class="row">
<div class="col-md-4 header">
<img src="http://www.mandroid.in/demo/srems/images/news_image_01.jpg" />
</div>
<div class="col-md-4 header">
<img src="http://www.mandroid.in/demo/srems/images/news_image_02.jpg" />
</div>
<div class="col-md-4 header">
<img src="http://www.mandroid.in/demo/srems/images/news_image_03.jpg" />
</div>
<div class="col-md-4 header">
<img src="http://www.mandroid.in/demo/srems/images/news_image_04.jpg" />
</div>
</div>
If you want to target individual headers...
Currently, every one of your elements has the class header, which is going to trigger the event on it's own, which may be what you are going for :
<div class="row">
<div class="col-md-4 header" >
<!-- Image omitted for brevity -->
</div>
<div class="col-md-4 header" >
<!-- Image omitted for brevity -->
</div>
<div class="col-md-4 header" >
<!-- Image omitted for brevity -->
</div>
<div class="col-md-4 header" >
<!-- Image omitted for brevity -->
</div>
</div>
This effect is magnified because each time a mouseover
or mouseleave
event occurs, it's animating every header
element :
// When any header is hovered over
$(".header").mouseleave(function () {
// Animate every header
$(".header").animate({ height: "50px" }, 600);
});
If you only want the animation to affect the header being hovered, simply change your inner animate selector to use $(this)
instead of $('.header')
:
$(".header").mouseleave(function () {
// Only animate the current element
$(this).animate({height: "50px" }, 600);
});
$(".header").mouseenter(function () {
// Only animate the current element
$(this).animate({ height: "100px"}, 600);
});
Example (Individual Child Headers)
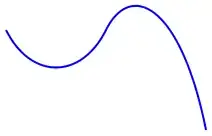
$(document).ready(function() {
$(".header").mouseleave(function() {
$(this).animate({
height: "50px"
}, 600);
});
$(".header").mouseenter(function() {
$(this).animate({
height: "100px"
}, 600);
});
})
body {
height: 1000px;
width: 100%;
background-color: #F0F0F0;
}
#header_div {
width: 100%;
height: 100px;
position: fixed;
top: 0;
left: 0;
}
#header_div img {
max-height: 100%;
max-width: 100%;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="row">
<div class="col-md-4 header">
<img src="http://www.mandroid.in/demo/srems/images/news_image_01.jpg" />
</div>
<div class="col-md-4 header">
<img src="http://www.mandroid.in/demo/srems/images/news_image_02.jpg" />
</div>
<div class="col-md-4 header">
<img src="http://www.mandroid.in/demo/srems/images/news_image_03.jpg" />
</div>
<div class="col-md-4 header">
<img src="http://www.mandroid.in/demo/srems/images/news_image_04.jpg" />
</div>
</div>