Probably the best and safest way is to write it manualy. If you are using vs2015 you can also copy the json and paste it as class.
You can find this option here: 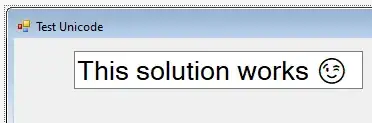
Another option is to use a website like http://json2csharp.com/ here you can paste the JSON and this will also generate classes for your JSON. Keep in mind that these generator are not 100% accurate so you might have to change some properties your self.
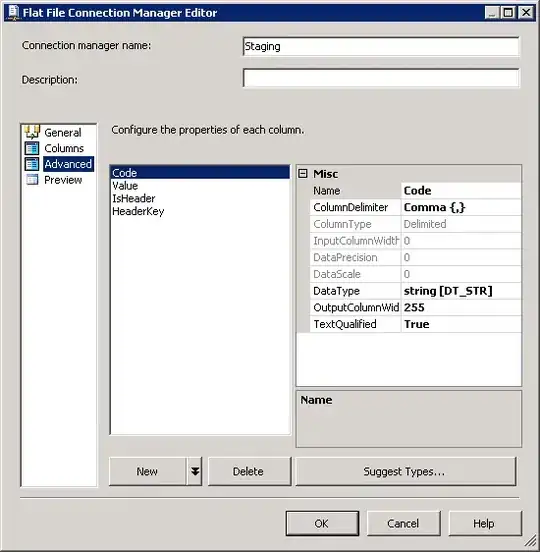
for you example this would look something like this:
public class MyClass
{
public List<string> rows { get; set; }
}
or with cells
public class RootObject
{
public List<List<string>> rows { get; set; }
}
Ones we got the model we can use a lib like JSON.net to deserialize our JSON into our model. On the website is also some extra documentation on how to use JSON.net
var model = JsonConvert.DeserializeObject<MyClass>(json); //json being the json string.
Test:
var c = new MyClass()
{
rows = new List<string>()
{
"string1",
"string2",
"string3"
}
};
Console.WriteLine(JsonConvert.SerializeObject(c));
Console.Read();
Result:
{
"rows": [
"string1",
"string2",
"string3"
]
}
With cell list
Test:
static void Main(string[] args)
{
var c = new MyClass()
{
rows = new List<List<string>>()
{
new List<string>() {"string1","string2","string3" }
}
};
Console.WriteLine(JsonConvert.SerializeObject(c));
Console.Read();
}
public class MyClass
{
public List<List<string>> rows { get; set; }
}
Result:
{
"rows": [
[
"string1",
"string2",
"string3"
]
]
}