This is my answer. Hope it help others. Extending HtmlTextView
gives us opportunity to add new attribute with string
format. Then, android:text
and custom:text2
can be concatenated programatically inside custom HtmlTextView
.
1. Extend HtmlTextView
or TextView if you are using it.
public class HtmlTextview extends HtmlTextView {
public HtmlTextview(Context context) {
super(context);
}
public HtmlTextview(Context context, AttributeSet attrs) {
super(context, attrs);
setupText(attrs);
}
public HtmlTextview(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
setupText(attrs);
}
private void setupText(AttributeSet attrs) {
String htmlString = (String) this.getText();
String text2;
TypedArray attributeValuesArray = getContext().obtainStyledAttributes(attrs, R.styleable.concatenate, 0, 0);
try{
text2 = attributeValuesArray.getString(R.styleable.concatenate_text2);
} finally {
attributeValuesArray.recycle();
}
if(text2 != null && text2.length() > 0){
htmlString = htmlString + text2;
this.setHtmlFromString(htmlString, new HtmlTextView.RemoteImageGetter());
}
}
}
2. HtmlTextview attributes
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="concatenate">
<attr name="text2" format="string"/>
</declare-styleable>
</resources>
3. In string.xml resources
<resources>
<string name="app_name">HtmlTry</string>
<string name="string_a">
<![CDATA[
<body>
<p>very long text. Can also formatted in <font color="#f44336">Html.</font></p>
</body>
]]>
</string>
<string name="string_b">
<![CDATA[
<body>
<p>This is string B</p>
</body>
]]>
</string>
</resources>
4. Lastly apply it inside xml layout.
<com.htmltry.HtmlTextview
android:id="@+id/tv"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/string_b"
custom:text2="@string/string_a"
/>
and the final result is:
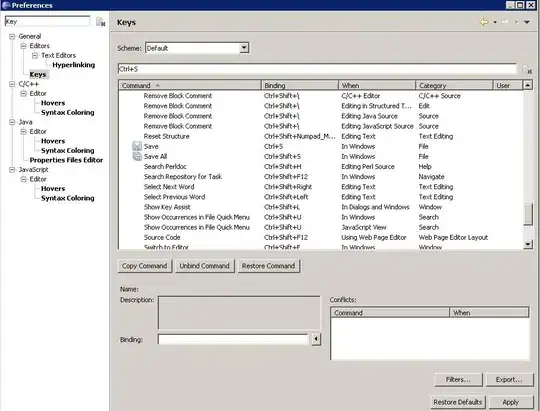