Description
^(?:(?=.*?[A-Z])(?:(?=.*?[0-9])(?=.*?[-!@#$%^&*()_[\]{},.<>+=])|(?=.*?[a-z])(?:(?=.*?[0-9])|(?=.*?[-!@#$%^&*()_[\]{},.<>+=])))|(?=.*?[a-z])(?=.*?[0-9])(?=.*?[-!@#$%^&*()_[\]{},.<>+=]))[A-Za-z0-9!@#$%^&*()_[\]{},.<>+=-]{7,50}$
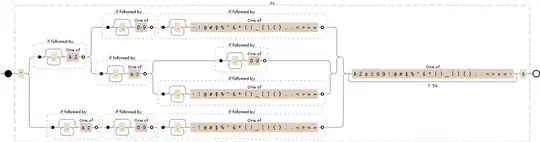
To see the image better, you can right click the image and select view in new window.
This regex will do the following
- Require the string to be 7 - 50 characters long
- Allow the string to be contain
A-Z
, a-z
, 0-9
, and !@#$%^&*()_[\]{},.<>+=-
characters
- Require at least one character from any three of the following cases
- English uppercase alphabet characters
A–Z
- English lowercase alphabet characters
a–z
- Base 10 digits
0–9
- Non-alphanumeric characters
!@#$%^&*()_[]{},.<>+=-
Example
Live Demo
https://regex101.com/r/jR9cC7/1
Sample Text
1 2 3 4 5 6
12345678901234567890123456789012345678901234567890124567890
aaaaAAAA1111
aaaaBBBBBBB
AAAAaaaa__
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa!A
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa!
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa!A
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa!AA
Allowed Strings
aaaaAAAA1111
AAAAaaaa__
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa!A
Explanation
NODE EXPLANATION
----------------------------------------------------------------------
^ the beginning of a "line"
----------------------------------------------------------------------
(?: group, but do not capture:
----------------------------------------------------------------------
(?= look ahead to see if there is:
----------------------------------------------------------------------
.*? any character except \n (0 or more
times (matching the least amount
possible))
----------------------------------------------------------------------
[A-Z] any character of: 'A' to 'Z'
----------------------------------------------------------------------
) end of look-ahead
----------------------------------------------------------------------
(?: group, but do not capture:
----------------------------------------------------------------------
(?= look ahead to see if there is:
----------------------------------------------------------------------
.*? any character except \n (0 or more
times (matching the least amount
possible))
----------------------------------------------------------------------
[0-9] any character of: '0' to '9'
----------------------------------------------------------------------
) end of look-ahead
----------------------------------------------------------------------
(?= look ahead to see if there is:
----------------------------------------------------------------------
.*? any character except \n (0 or more
times (matching the least amount
possible))
----------------------------------------------------------------------
[- any character of: '-', '!', '@',
!@#$%^&*()_[\] '#', '$', '%', '^', '&', '*', '(',
{},.<>+=] ')', '_', '[', '\]', '{', '}', ',',
'.', '<', '>', '+', '='
----------------------------------------------------------------------
) end of look-ahead
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
(?= look ahead to see if there is:
----------------------------------------------------------------------
.*? any character except \n (0 or more
times (matching the least amount
possible))
----------------------------------------------------------------------
[a-z] any character of: 'a' to 'z'
----------------------------------------------------------------------
) end of look-ahead
----------------------------------------------------------------------
(?: group, but do not capture:
----------------------------------------------------------------------
(?= look ahead to see if there is:
----------------------------------------------------------------------
.*? any character except \n (0 or more
times (matching the least amount
possible))
----------------------------------------------------------------------
[0-9] any character of: '0' to '9'
----------------------------------------------------------------------
) end of look-ahead
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
(?= look ahead to see if there is:
----------------------------------------------------------------------
.*? any character except \n (0 or more
times (matching the least amount
possible))
----------------------------------------------------------------------
[- any character of: '-', '!', '@',
!@#$%^&*()_[ '#', '$', '%', '^', '&', '*', '(',
\]{},.<>+=] ')', '_', '[', '\]', '{', '}',
',', '.', '<', '>', '+', '='
----------------------------------------------------------------------
) end of look-ahead
----------------------------------------------------------------------
) end of grouping
----------------------------------------------------------------------
) end of grouping
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
(?= look ahead to see if there is:
----------------------------------------------------------------------
.*? any character except \n (0 or more
times (matching the least amount
possible))
----------------------------------------------------------------------
[a-z] any character of: 'a' to 'z'
----------------------------------------------------------------------
) end of look-ahead
----------------------------------------------------------------------
(?= look ahead to see if there is:
----------------------------------------------------------------------
.*? any character except \n (0 or more
times (matching the least amount
possible))
----------------------------------------------------------------------
[0-9] any character of: '0' to '9'
----------------------------------------------------------------------
) end of look-ahead
----------------------------------------------------------------------
(?= look ahead to see if there is:
----------------------------------------------------------------------
.*? any character except \n (0 or more
times (matching the least amount
possible))
----------------------------------------------------------------------
[- any character of: '-', '!', '@', '#',
!@#$%^&*()_[\]{} '$', '%', '^', '&', '*', '(', ')',
,.<>+=] '_', '[', '\]', '{', '}', ',', '.',
'<', '>', '+', '='
----------------------------------------------------------------------
) end of look-ahead
----------------------------------------------------------------------
) end of grouping
----------------------------------------------------------------------
[A-Za-z0- any character of: 'A' to 'Z', 'a' to 'z',
9!@#$%^&*()_[\]{},.< '0' to '9', '!', '@', '#', '$', '%', '^',
>+=-]{7,50} '&', '*', '(', ')', '_', '[', '\]', '{',
'}', ',', '.', '<', '>', '+', '=', '-'
(between 7 and 50 times (matching the most
amount possible))
----------------------------------------------------------------------
$ before an optional \n, and the end of a
"line"
----------------------------------------------------------------------