it's obvious you can't but you can
- you can assign
null
to object you wanna free it from GC
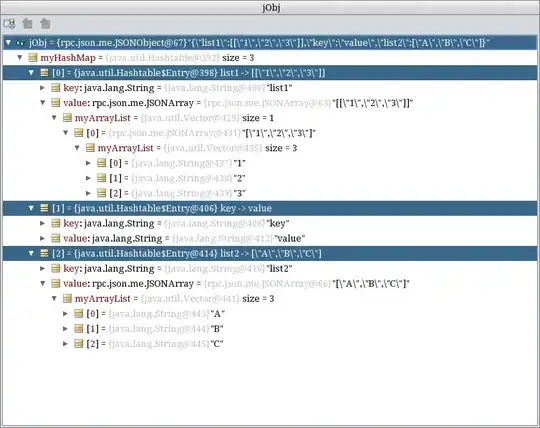
- you can use
WeakReferences
:
A weak reference, simply put, is a reference that isn't strong enough
to force an object to remain in memory. Weak references allow you to
leverage the garbage collector's ability to determine reachability for
you, so you don't have to do it yourself. You create a weak reference
like this:
WeakReference weakWidget = new WeakReference(widget); and then
elsewhere in the code you can use weakWidget.get() to get the actual
Widget object. Of course the weak reference isn't strong enough to
prevent garbage collection, so you may find (if there are no strong
references to the widget) that weakWidget.get() suddenly starts
returning null.
If GC
finds that an object is weakly reachable (reachable only through weak references), it'll clear the weak references to that object immediately
.
HashMap<StringBuilder, Integer> map = new HashMap<>();
WeakReference<HashMap<StringBuilder, Integer>> aMap = new WeakReference<>(map);
map = null;
while (null != aMap.get()) {
aMap.get().put(new StringBuilder("abbas"),
new Integer(123));
System.out.println("Size of aMap " + aMap.get().size());
System.gc();
}
System.out.println("Its garbage collected");
//Size of aMap 1
//Its garbage collected
and in a general way you create Object
with new
but you have no power to free it or run the GC
.