Checking Scope
Consider placing your <script>
tag at the end of your code (just prior to the </body>
element or at least somewhere after your <div>
) to ensure that it can see the element it needs to target :
<div id="txt"></div>
<script>
// Your code here
</script>
This will ensure that your document.getElementById()
function can "see" the element you are targeting and access it accordingly when needed.
Concatenate Your Values
While you absolutely could change your =
to a +=
within your loop to continually concatenate to your contents :
document.getElementById("txt").innerHTML + = arr[i];
A better approach as mentioned by 4castle in his comment, would be to build a string within the loop and then set it after the final iteration to avoid issues with actually appending to the innerHTML
property, which can cause some unexpected DOM issues :
var arr = new Array("pro","pro1","pro2");
var content = '';
for(var i =0 ;i<arr.length;i++){
// Build your content
content += arr[i];
}
// Now set it
document.getElementById("txt").innerHTML = content;
Avoid Looping with Array.join()
A better approach still would be to avoid the loop alltogether and to use the Array.join()
function to concatenate and set your text without a loop :
var arr = new Array("pro","pro1","pro2");
document.getElementById("txt").innerHTML = arr.join('');
Example
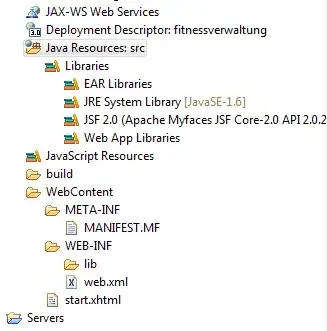
<pre>Setting with Content</pre>
<div id="txt"></div>
<pre>Setting via Array.join()</pre>
<div id="txt2"></div>
<pre>document.write()</pre>
<script>
var arr = new Array("pro", "pro1", "pro2");
var content = '';
for (var i = 0; i < arr.length; i++) {
content += arr[i];
document.write(arr[i]);
}
document.getElementById('txt').innerHTML = content;
document.getElementById('txt2').innerHTML = arr.join('');
</script>