You can first verify if this setting in Eclipse is enabled.
Window -> Preferences -> Java -> Debug -> Suspend execution on uncaught exceptions
If this setting is enabled, any uncaught exception will suspend the JVM exactly at the point its thrown, including classes invoked using reflection. This is without adding any breakpoint, but provided its unhandled, i.e. your code is not even invoked by an external code from a try-catch.
For e.g.
int a = 0, b= 0;
System.out.println(a/b); // ArithmeticException
Even if this code is called from a reflection invoked code, eclipse will suspend at sysout with all variables still available on the stack.
However in Android's startup class ZygoteInit
there is this line :
catch (Throwable t) {
Log.e(TAG, "Error preloading " + line + ".", t);
if (t instanceof Error) {
throw (Error) t;
}
if (t instanceof RuntimeException) {
throw (RuntimeException) t;
}
throw new RuntimeException(t);
}
The reason why such code would break Eclipse debugging is, the RuntimeException
is now no more unhandled. Your UncaughtExceptionHandler
may actually be catching the startup class instead of your user code. This is for regular Eclipse.
Solution 1 :
- Goto Run -> Add Java Exception Breakpoint ->
Throwable
- Click on
Throwable
in the Breakpoint view
- Right click -> Breakpoint properties -> Add package -> OK
- Check on the option Subclasses of this exception
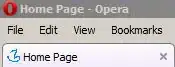
Note : This can marginally catch a java.lang.OutOfMemoryError
but definitely cannot catch a java.lang.StackOverflowError
.
Solution 2 : (Only if too many caught exceptions, NOT recommended otherwise)
- Copy the source code of
com.android.internal.os.ZygoteInit
to a new project say MyBootstrap
Modify the catch (Throwable t)
block to catch only Error
} catch (Error t) {
Log.e(TAG, "Error preloading " + line + ".", t);
throw t;
}
Go-to debug configurations -> Classpath -> Click Bootstrap Entries -> Add projects -> MyBootstrap
. Move this project to the top
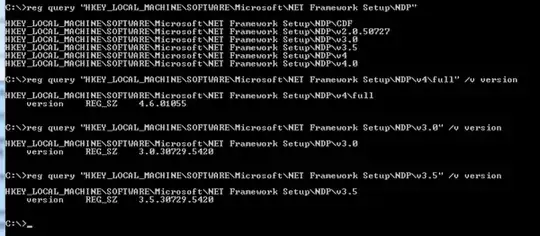