You could do it in more sophisticated way using LinearLayout as a button.
The simple example:
XML file with the LinearLayout button:
<LinearLayout android:id="@+id/sophisticated_button"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="150dp">
<LinearLayout
android:background="#333333"
android:layout_width="0dp"
android:layout_height="match_parent"
android:gravity="center"
android:layout_weight="1">
<ImageView
android:layout_width="66dp"
android:layout_height="66dp"
android:src="@drawable/present"/>
</LinearLayout>
<TextView
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="2"
android:gravity="center"
android:text="SEND A GIFT \n TO A FRIEND"/>
</LinearLayout>
where present represents the Image located in your drawable directory.
The Activity where the button is located looks like this:
public class MainActivity extends Activity implements View.OnClickListener {
private LinearLayout buttonLinearLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.skuska);
buttonLinearLayout = (LinearLayout)findViewById(R.id.sophisticated_button);
buttonLinearLayout.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.sophisticated_button:
Toast.makeText(getApplicationContext(), "Sophisticated Button Pressed", Toast.LENGTH_LONG).show();
break;
}
}
}
And the output:
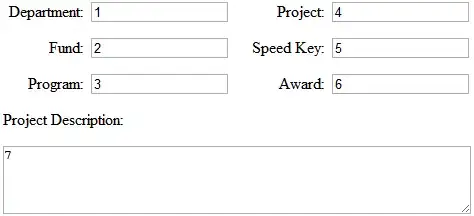