Overview Map
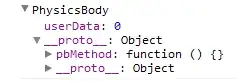
bucket details of map[string]string
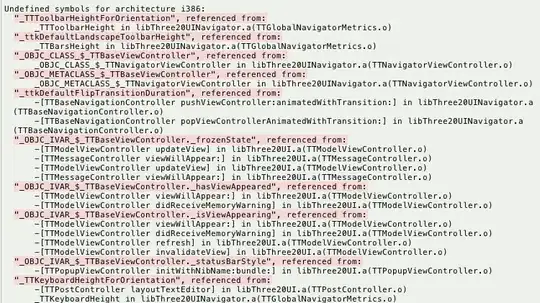
Each bucket stores
- an array of hash codes
- The array of hash codes stores the hash code for each key in the bucket, this is used for faster comparison.
- a list of key-value pairs
- Each bucket can store a maximum of 8 such key-value pairs.
- an overflow pointer.
- The overflow pointer can point to a new bucket if a bucket receives more than 8 values.
What happens when we insert a new value in the map
Hash function is called and a hash code is generated for the given key, based on a part of the hash code a bucket is determined to store the key-value pair. Once the bucket is selected the entry needs to be held in that bucket. The complete hash code of the incoming key is compared with all the hashes from the initial array of hash codes i.e h1, h2... if no hash code matches that means this is a new entry. Now if the bucket contains an empty slot then the new entry is stored at the end of the list of key-value pairs, else a new bucket is created and the entrance is stored in the new bucket, and the overflow pointer of the old bucket points to this new bucket.
What happens when the map grows
Every time the number of elements in a bucket reaches a certain limit, i.e the load factor which is 6.5, the map will grow in size by doubling the number of buckets. This is done by creating a new bucket array consisting of twice the number of buckets than the old array, and then copying all the buckets from the old to a new array but this is done very efficiently over time and not at once. During this, the map maintains a pointer to the old bucket which is stored at the end of the new bucket array.
What happens when we delete an entry from the map
Maps in golang only grow in size. Even if we delete all the entries from the map, the number of buckets will remain the same
Source: