REMOTE_HOST usually contains the result of a reverse dns lookup and can also be done in PHP using gethostbyaddr in the case, that your server does not fill this environment variable. It gets derived from the $_SERVER['REMOTE_ADDR']
value, which represents the (IP)-address as its name suggests.
Banning IP-Addresses on a shared host is not optimal and I will come to that later. Assuming you are using a shared host I would not let the script die like you did. Instead I would just return a HTTP header in order to (at least) save some bandwidth on that IP, like:
if($_SERVER['REMOTE_ADDR'] == "127.0.0.1")
{ header("HTTP/1.1 403 Forbidden" ); exit; }
and returns something like this to your visitor (using chromium):
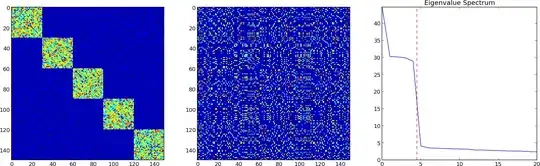
A cleaner and more professional approach to block IP addresses is not possible on many shared hosts, but should be mentioned here anyways, because it saves bandwidth, memory and cpu-cycles and can be described as dynamic creation of firewall rules. There are tools like fail2ban helping to overcome compatibility issues between different firewalls keeping your PHP application portable between root servers. Fail2ban can scan all kinds of log files, even custom ones. Your PHP application could just write to a log file and fail2ban would disallow any connection attempt from that IP address to your server. Sounds cool? Root servers ain't expensive nowadays if you would like to try it.