To connect the points, use geom_line
(if that doesn't give you what you need, please explain what you're trying to accomplish).
I haven't used geom_encircle
, but another option is to use a filled marker with the fill deleted to create the circles. Here's an example, using the built-in mtcars
data frame for illustration:
ggplot(mtcars, aes(wt, mpg)) +
geom_point() +
geom_point(data=mtcars[mtcars$mpg>30,],
pch=21, fill=NA, size=4, colour="red", stroke=1) +
theme_bw()
pch=21
is one of the filled markers (see ?pch
for more info on other available point markers). We set fill=NA
to remove the fill. stroke
sets the thickness of the circle border.
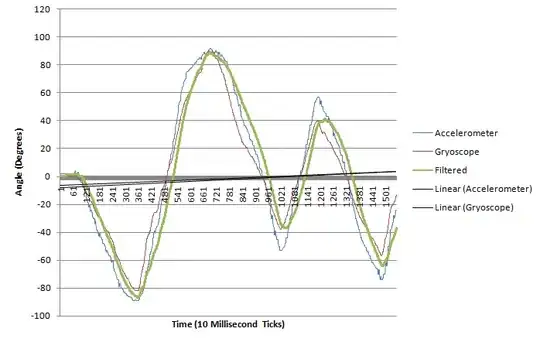
UPDATE: To add a line to this chart, using the example above:
ggplot(mtcars, aes(wt, mpg)) +
geom_line() +
geom_point() +
geom_point(data=mtcars[mtcars$mpg>30,],
pch=21, fill=NA, size=4, colour="red", stroke=1) +
theme_bw()
However, if (as in my original code for this graph) you put the aes
statement inside the geom
, rather than in the initial call to ggplot
, then you need to include an aes
statement inside geom_line
as well.