This is sort of totally wrong.
It's very common for experienced developers to not really understand that Unity is not object oriented, and has utterly no connection to concepts like inheritance.
(Sure, the programming language currently used for writing components in Unity, happens to be OO, but that's largely irrelevant.)
Good news though, the solution is incredibly simple.
All you do in Unity is write behaviors that do what you want.
Essay about it ... https://stackoverflow.com/a/37243035/294884
Try to get this concept: say you have a "robot attack device" in your game.
So, the only thing in Unity scenes is GameObjects. (There is nothing else - at all.)
Your "robot attack device" would have these behaviors. ("Behaviours" meaning components attached to it. Components are MonoBehavior.)
"robot attack device"
• animate Z position
• shoot every ten seconds
• respond to bashes with sound effect
and so on.
Whereas, your "kawaii flower" might have these behaviors
"kawaii flower fixed NPC"
• shoot every 5 seconds
• respond to bashes with sound effect
• rainbow animation
In this case you're asking how to write a "animate Y" behavior.
It's very easy, first note that everything you do in Unity you do with an extension, so it will be like:
public static void ForceY( this Transform tt, float goY )
{
Vector3 p = tt.position;
p.y = goY;
tt.position = p;
}
Quick tutorial on extensions: https://stackoverflow.com/a/35629303/294884
And then regarding the trivial component (all components are trivial) to animate Y, it's just something like
public class AlwaysMoveOnYTowards:MonoBehaviour
{
[System.NonSerialized] public float targetY;
void Update()
{
float nextY = .. lerp, or whatever, towards targetY;
transform.ForceY(nexyT);
}
}
Note that, of course, you just turn that component on and off as needed. So if the thing is asleep or whatever in your game
thatThing.GetComponent<AlwaysMoveOnYTowards>().enabled = false;
and later true
when you want that behavior again.
Recall, all you're doing is making a model for each thing in your game (LaraCroft, dinosaur, kawaiiFlower, bullet .. whatever).
(Recall there are two ways to use models in Unity, either use Unity's prefab system (which some like, some don't) or just have the model sitting off-screen and then Instantiate or use as needs be.)
(Note that a recent confusion in Unity (to choose one) is: until some years ago you had to write your own pooling for things that you had a few of. Those days are long gone, never do pooling now. Just Instantiate. A huge problem with Unity is totally out-of-date example code sitting around.)
Incidentally, here's the same sort of ForceY in the case of the UI system
public static void ForceYness(this RectTransform rt, float newY)
{
Vector2 ap = rt.anchoredPosition;
ap.y = newY;
rt.anchoredPosition = ap;
}
Finally if you just want to animate something on Y to somewhere one time, you should get in to the amazing Tweeng which is the crack cocaine of game engineering:
https://stackoverflow.com/a/37228628/294884
A huge problem with Unity is folks often don't realize how simple it is to make games. (There are many classic examples of this: you get 1000s of questions asking how to make a (totally trivial) timer in Unity, where the OP has tied themselves in knots using coroutines. Of course, you simply use Invoke
or InvokeRepeating
99% of the time for timers in Unity. Timers are one of the most basic parts of a game engine: of course, obviously Unity threw in a trivial way to do it.)
Here's a "folder" (actually just a pointless empty game object, with these models sitting under it) holding some models in a scene for a game.
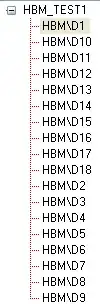
Those are some of the enemies in the game. As an engineer I just popped them in there, the game "designer" would come along and set the qualities (so, the potato is fast, the turnip is actually an unkillable boss, the flying head connects to Google Maps or whatever).
Same deal, this is from the "folder" (aside, if you use an empty game object as a, well, folder to just hold things, it's often referred to as a "folder" - it's no less a game object) with some "peeps" ("player projectiles"). Again these are the very models of these things to be used in the game. So obviously these would just be sitting off-screen, as you do with games. Look, here they are, literally just sitting around an "-10" or something outside of the camera frustrum (a good place to remember a Unity "camera" is nothing more than ............. a GameObject
with certain components (which Unity already wrote for our convenience) attached.)
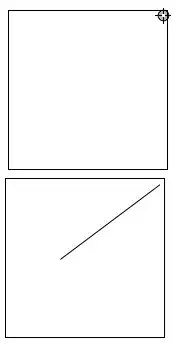
(Again: in many cases you may prefer to use prefabs. Unity offers both approaches: prefabs, or "just sitting off-screen". Personally I suggest there is a lot to be said for "just sitting off-screen" at first, as it makes you engineer proper states and so on; you'll inherently have to have a "just waiting" state and so on, which is highly important. I strongly encourage you, at first, to just 'sit your models around off-screen', don't use prefabs at first. Anyway that's another issue.)

Here then are indeed some of those peeps ...
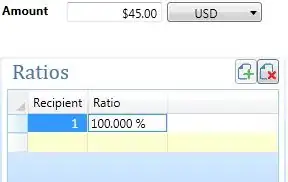
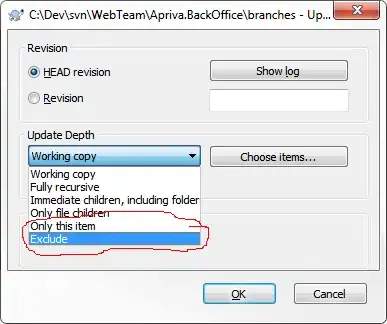
The "game designer" comes along and sets that sort of thing as they see fit. (And of course, adds the profoundly important Component
s such as, you know, "the renderer", sound effects, and the like).
Note: you may notice above: because "life's like that" in the naming there (it's purely a naming issue, not a deep one) we very un-sensibly went against just what I describe here. So, notice I have a component that should be named, say, "Killableness" or perhaps just "projectileness" or indeed just "power" or "speed". Just because life's like that, I rather unsensibly named it "missile" or "projectile" rather than indeed "flight" or "attackPower". You can see this is very very bad because when I reuse the component "attackPower" (stupidly named here "missile") in the next project, which involves say robotic diggers or something rather than missiles, everyone will scream at me "Dude why is our attack power component, attached to our robotic spiders, called 'missile' instead of 'attack power', WTF?" I'm sure you see what I mean.
Note too, there's a great example there of, while Unity and GameObject
have no connection at all to computer science or programming, it's totally normal - if confusing - that in writing components you have to be a master of OO since (as it happens) the current language used by Unity does indeed happen to be an OO language (but do bear in mind they could change to using Lisp or something at any time, and it wouldn't fundamentally affect Unity. As an experienced programmer you will instantly see that (for the components discussed here) I have something like a base class "Flightyness" and then there are subclasses like "ParabolaLikeFlightyness" "StraightlineFlightyness" "BirdFlightyness" "NonColliderBasedFlightyness" "CrowdNetworkDrivenFlightyness" "AIFlightyness" and so on; you can see each have the "general" settings (for Steve the game designer to adjust) and more specific settings (again for Steve to adjust). Some random code fragments that will make perfect sense to you...
public class Enemy:BaseFrite
{
public tk2dSpriteAnimator animMain;
public string usualAnimName;
[System.NonSerialized] public Enemies boss;
[Header("For this particular enemy class...")]
public float typeSpeedFactor;
public int typeStrength;
public int value;
public class Missile:Projectile
{
[Header("For the missile in particular...")]
public float splashMeasuredInEnemyHeight;
public int damageSplashMode;
Note the use of [Header
... one of the most important things in Unity!
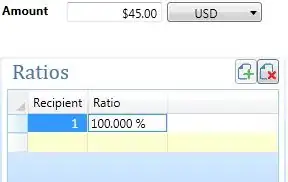
Note how good the "Header" thing works, especially when you chain down through derives. It's nothing but pleasure working on a big project where everyone works like that, making super-tidy, super-clear Inspector panels for your models sitting off-screen. It's a case of "Unity got it really right".