I will try to answer your question, but you really don't provide enough detail about your code for me to be sure my answer will help you.
My best guess is that your Clinic
class looks something like this:
public class Clinic {
public String details;
public void details(String input) {
details = input;
}
}
The first thing I notice is that your call to JOptionPane.showInputDialog
does not appear to be doing what you want. Again, I'm only guessing as to what you want based on what little information is provided. I'm thinking you want a dialog that asks the user for one of two inputs - either P for "Proceed" or D for "Done". If you look at the Javadoc for JoptionPane
you will see that your code is invoking this method which is causing your dialog to appear like this:

Now, if you simply click OK to this dialog and your Clinic
class is similar to the one I show above, then the details
field is set to " [D]one" which, obviously, is not equal to "D" and therefore your loop never ends.
If you change the line that calls the details
method to this:
c.details(JOptionPane.showInputDialog("[P]roceed\n[D]one","D"));
You will get what you want - the prompt will look like this:
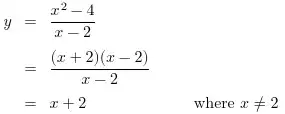
and when you press OK the loop will end.
Some things to consider regarding your code:
- The call to
JOptionPane.showInputDialog
returns the text entered by the user. If the user presses Cancel you will get a java.lang.NullPointerException when the loop attempts to access c.details (again, assuming Clinic
works as I outlined above)
- In general you should always write your
equals
comparison using the constant first and the variable second so that you avoid any possibility of NullPointerException. For example in your code: }while(!c.details.equals("D"));
would change to }while(!"D".equals(c.details));
- You should generally never make the fields of a class public. Giving direct access to the internal data of objects can make your code harder to maintain (as @Roland mentioned in the comments). Instead use getter and setter methods. The accepted answer to this question explains this in more detail.