What will MyNumber br 6 or 8 ?
It will be 8. When the following line would be executed:
var o = new MyObject {MyNumber = 8 };
an object of type MyObject
would be created and afterwards the value of MyNumber
would be set to 8. Under the hood the default constructor would be used for the creation of the object. So before MyNumber
would be set to 8, it would be 6.
and whats the story with the 7 i just added?
It is the same as for the above case. Now, you are in the case of an auto property initializer (a feature that has been introduced in C# 6). The following
public int MyNumber { get; set; } = 7;
is equivalent as to initialize the value of MyNumber
inside the default constructor.
If you create a console application with the following code:
public class MyObject
{
public MyObject()
{
MyNumber = 6;
}
public int MyNumber { get; set; }
}
class Program
{
static void Main(string[] args)
{
var o = new MyObject { MyNumber = 8 };
}
}
and then compile the code and decompile it using ildasm, you will get the following picture, if you look at the IL code of Main.
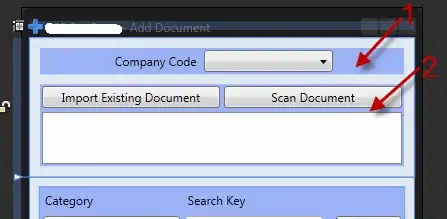
From the first underlined line it's clear that the default object initializer is called. Then at the second underline line the value of a 8 is set to the corresponding backing field.
Furthermore, If you compile the code for the following console app:
public class MyObject
{
public int MyNumber { get; set; } = 8;
}
class Program
{
static void Main(string[] args)
{
var o = new MyObject { MyNumber = 8 };
}
}
you will get exactly the same IL code !