As noted in the comments, it is not a trivial task display UTF-8 Arabic text correctly on an embedded device. You need to handle text direction, joining and character encoding.
I've had an attempt at this in the past for a PHP ESC/POS driver that I maintain, and was unable to get joined Arabic characters in native ESC/POS. However, I did end up settling on this workaround (PHP) that printed images instead.
The basic steps to working around this are:
- Get an Arabic font, some text libraries, and an image library
- Join ('reshape') the characters
- Convert the UTF-8 to LTR (print) order, using the bidi text layout algorithm
- Slap it on an image, right aligned
- Print the image
To port this to python, I lent on this answer using Wand. The Python Image Library (PIL) was displaying diacritics as separate characters, making the output unsuitable.
The dependencies are listed in the comments.
#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Print an Arabic string to a printer.
# Based on example from escpos-php
# Dependencies-
# - pip install wand python-bidi python-escpos
# - sudo apt-get install fonts-hosny-thabit
# - download arabic_reshaper and place in arabic_reshaper/ subfolder
import arabic_reshaper
from escpos import printer
from bidi.algorithm import get_display
from wand.image import Image as wImage
from wand.drawing import Drawing as wDrawing
from wand.color import Color as wColor
# Some variables
fontPath = "/usr/share/fonts/opentype/fonts-hosny-thabit/Thabit.ttf"
textUtf8 = u"بعض النصوص من جوجل ترجمة"
tmpImage = 'my-text.png'
printFile = "/dev/usb/lp0"
printWidth = 550
# Get the characters in order
textReshaped = arabic_reshaper.reshape(textUtf8)
textDisplay = get_display(textReshaped)
# PIL can't do this correctly, need to use 'wand'.
# Based on
# https://stackoverflow.com/questions/5732408/printing-bidi-text-to-an-image
im = wImage(width=printWidth, height=36, background=wColor('#ffffff'))
draw = wDrawing()
draw.text_alignment = 'right';
draw.text_antialias = False
draw.text_encoding = 'utf-8'
draw.text_kerning = 0.0
draw.font = fontPath
draw.font_size = 36
draw.text(printWidth, 22, textDisplay)
draw(im)
im.save(filename=tmpImage)
# Print an image with your printer library
printer = printer.File(printFile)
printer.set(align="right")
printer.image(tmpImage)
printer.cut()
Running the script gives you a PNG, and prints the same to a printer at "/dev/usb/lp0".
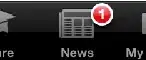
This is a standalone python-escpos demo, but I'm assuming that Odoo has similar commands for alignment and image output.
Disclaimer: I don't speak or write Arabic even slightly, so I can't be sure this is correct. I'm just visually comparing the print-out to what Google translate gave me.
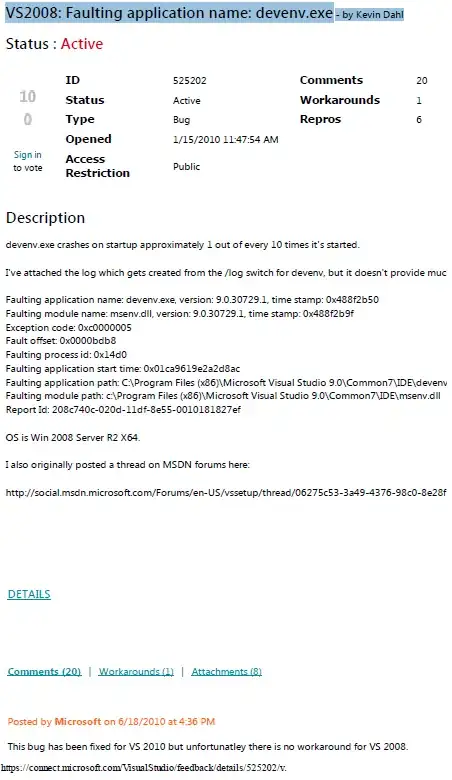