As Naga Sai A pointed out, you shouldn't have duplicate id values in your HTML document. So make sure that each input has a unique id.
If I understood you correctly, you don't need to use different values for each row data in your case. You need different names for them only:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ page import="java.util.List" %>
<%@ page import="java.util.Arrays" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>Change Data</title>
</head>
<body>
<%!
public class Data {
private int id;
public Data(int id) {
this.id = id;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
List<Data> dataList = Arrays.asList(new Data(10), new Data(11), new Data(12));
%>
<% request.setAttribute("dataList", dataList); %>
<c:url var="controller" value="/controller"/>
<p>Choose your actions for data</p>
<form action="${controller}" method="POST">
<table>
<thead>
<tr>
<td>insert</td>
<td>update</td>
<td>delete</td>
</tr>
</thead>
<tbody>
<c:forEach var="data" items="${dataList}">
<tr>
<td>
<label for="insert-${data.id}">Insert ${data.id}: </label>
<input type="radio" name="recordIds${data.id}" value="insert" id="insert-${data.id}"/>
</td>
<td>
<label for="update-${data.id}">Update ${data.id}: </label>
<input type="radio" name="recordIds${data.id}" value="update" id="update-${data.id}"/>
</td>
<td>
<label for="delete-${data.id}">Delete ${data.id}: </label>
<input type="radio" name="recordIds${data.id}" value="delete" id="delete-${data.id}"/>
</td>
</tr>
</c:forEach>
</tbody>
</table>
<input type="submit" value="Submit actions">
</form>
</body>
</html>
will give you a form
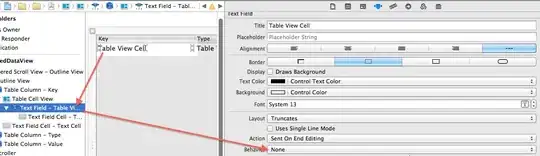
that will redirect its input to the servlet with doPost() method
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
Collections.list(request.getParameterNames()).forEach(paramName -> {
if (paramName.startsWith("recordIds")) {
String id = paramName.substring(9, paramName.length());
String action = request.getParameter(paramName);
try {
response.getWriter().println("Selected action for data with id=" + id + " is " + action);
} catch (IOException ex) {
ex.printStackTrace();
}
}
});
}
which prints the result
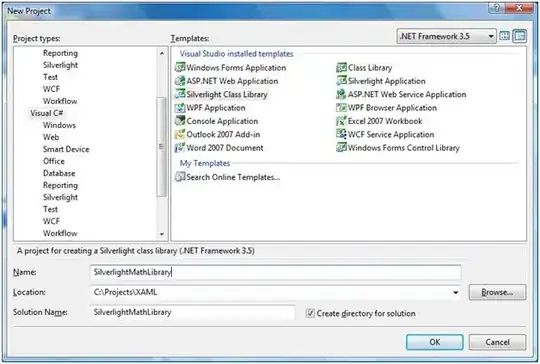
Ps: don't define Data class like this, the use of scriplets is highly discouraged, you should create your Data class separately and try to avoid Java code in JSP, i used it for demonstration purposes only