While this, as other answers have pointed out, is not a problem due to the dynamic typing, in fact, for Python3, this is a very real issue when it comes to type annotations. And this will not work (note a type annotation of the method argument):
class A:
def do_something_with_other_instance_of_a(self, other: A):
print(type(other).__name__)
instance = A()
other_instance = A()
instance.do_something_with_other_instance_of_a(other_instance)
results in:
def do_something_with_other_instance_of_a(self, other: A):
NameError: name 'A' is not defined
more on the nature of a problem here:
https://www.python.org/dev/peps/pep-0484/#the-problem-of-forward-declarations
You can use string literals to avoid forward references

Other way is NOT using python3-style type annotation in such cases,
and this is the only way if you have to keep your code compatible with earlier versions of Python.
Instead, for the sake of getting autocompletion in my IDE (PyCharm), you can docstrings like this:
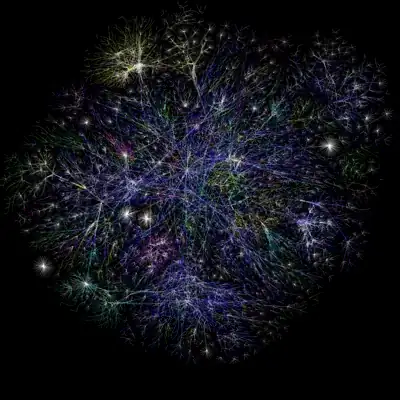
Update:
alternatively, instead of using docstrings, you can use "type: " annotations in a comment. This will also ensure that mypy static type checking will work (mypy doesn't seem to care about docstrings):
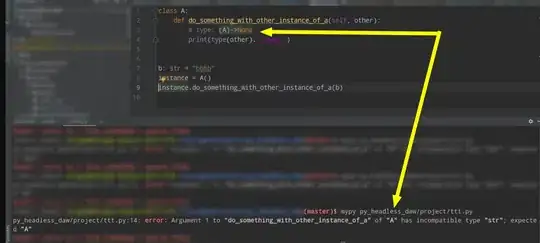