A very simple chat model code, you can take a look:
#import "ViewController.h"
@interface ViewController ()
@property UIView* containerView;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
_containerView = [[UIView alloc] initWithFrame:[UIScreen mainScreen].bounds];
UITableView* tableView = [[UITableView alloc] initWithFrame:CGRectMake(0, 0, [UIScreen mainScreen].bounds.size.width, [UIScreen mainScreen].bounds.size.height-30)];
UITextField* tfInput = [[UITextField alloc] initWithFrame:CGRectMake(0, tableView.frame.size.height, [UIScreen mainScreen].bounds.size.width-50, 30)];
tfInput.backgroundColor = [UIColor grayColor];
UIButton* btnSend = [[UIButton alloc] initWithFrame:CGRectMake(tfInput.frame.size.width, tfInput.frame.origin.y, 50, 30)];
[btnSend setTitle:@"Send" forState:UIControlStateNormal];
[btnSend setTitleColor:[UIColor blueColor] forState:UIControlStateNormal];
[btnSend addTarget:self action:@selector(btnClicked) forControlEvents:UIControlEventTouchUpInside];
[_containerView addSubview:tableView];
[_containerView addSubview:tfInput];
[_containerView addSubview:btnSend];
[self.view addSubview:_containerView];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyboardWillShow:) name:UIKeyboardWillShowNotification object:nil];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyboardWillHide:) name:UIKeyboardWillHideNotification object:nil];
}
- (void)keyboardWillShow:(NSNotification *)notification {
NSDictionary *userInfo = [notification userInfo];
NSValue* aValue = [userInfo objectForKey:UIKeyboardFrameEndUserInfoKey];
float keyboardHeight = [aValue CGRectValue].size.height;
//Resize the container
_containerView.frame = CGRectMake(0, - keyboardHeight, [UIScreen mainScreen].bounds.size.width, [UIScreen mainScreen].bounds.size.height);
}
-(void)btnClicked{
[self.view endEditing:YES];
}
- (void)keyboardWillHide:(NSNotification *)notification {
_containerView.frame = [UIScreen mainScreen].bounds;
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
This is a screenshot for using storyboard:
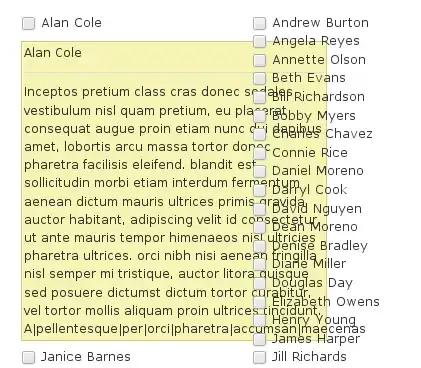