In asp.net framework Web API , I was able to add the Bearer token on the UI and make it work properly by two different ways.
Way 1:
Adding an operation fitler. Create the following class :
public class AuthorizationHeaderParameterOperationFilter : IOperationFilter
{
public void Apply(Operation operation, SchemaRegistry schemaRegistry, ApiDescription apiDescription)
{
if (operation.parameters == null)
{
operation.parameters = new List<Parameter>();
}
operation.parameters.Add(new Parameter
{
name = "Authorization",
@in = "header",
description = "access token",
required = false,
type = "string",
@default = "Bearer "
});
}
}
and now in the SwaggerConfig.cs add the following:
GlobalConfiguration.Configuration
.EnableSwagger(c =>
{
// other settings
c.OperationFilter<AuthorizationHeaderParameterOperationFilter>();
})
.EnableSwaggerUi(c =>
{
// UI configurations
});
Way 2:
We can use the DocumentFilter as well to iterate all the operation and add the header, in the following one we skip the operation which actually takes username and password and gives the token for the first time:
public class SwaggerPathDescriptionFilter : IDocumentFilter
{
private string tokenUrlRoute = "Auth";
// the above is the action which returns token against valid credentials
private Dictionary<HeaderType, Parameter> headerDictionary;
private enum HeaderType { TokenAuth };
public void Apply(SwaggerDocument swaggerDoc, SchemaRegistry schemaRegistry, IApiExplorer apiExplorer)
{
CreateHeadersDict();
var allOtherPaths = swaggerDoc.paths.Where(entry => !entry.Key.Contains(tokenUrlRoute)) //get the other paths which expose API resources and require token auth
.Select(entry => entry.Value)
.ToList();
foreach (var path in allOtherPaths)
{
AddHeadersToPath(path, HeaderType.TokenAuth);
}
}
/// <summary>
/// Adds the desired header descriptions to the path's parameter list
/// </summary>
private void AddHeadersToPath(PathItem path, params HeaderType[] headerTypes)
{
if (path.parameters != null)
{
path.parameters.Clear();
}
else
{
path.parameters = new List<Parameter>();
}
foreach (var type in headerTypes)
{
path.parameters.Add(headerDictionary[type]);
}
}
/// <summary>
/// Creates a dictionary containin all header descriptions
/// </summary>
private void CreateHeadersDict()
{
headerDictionary = new Dictionary<HeaderType, Parameter>();
headerDictionary.Add(HeaderType.TokenAuth, new Parameter() //token auth header
{
name = "Authorization",
@in = "header",
type = "string",
description = "Token Auth.",
required = true,
@default = "Bearer "
});
}
}
and then we need to regiter it in the SwaggerConfig.cs :
GlobalConfiguration.Configuration
.EnableSwagger(c =>
{
// other settings
c.DocumentFilter<SwaggerPathDescriptionFilter>();
})
.EnableSwaggerUi(c =>
{
// UI configurations
});
Now we will see the Token input for the headers in the swagger UI like:
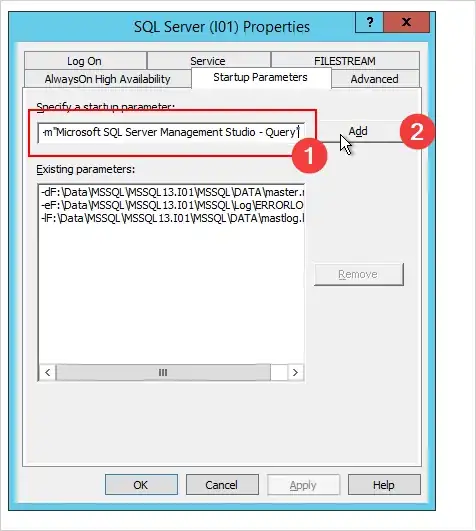