If I understand correctly you want to segue to another ViewController when your users press on an UIImageView. So here is my solution using an UITapGestureRecognizer (put this code in viewDidLoad):
yourImageView.isUserInteractionEnabled = true
let yourImageTapGesture = UITapGestureRecognizer(target: self, action: #selector(ViewController.test(_ :)))
yourImageTapGesture.delegate = self
yourImageTapGesture.numberOfTapsRequired = 1
yourImageView.addGestureRecognizer(yourImageTapGesture)
Then in your ViewController you have to make the function test, which is triggered when somebody taps on your UIImageView:
func test(_ sender: AnyObject) {
self.performSegue(withIdentifier: "YourSegueName",sender: self)
}
Important: "YourSegueName" is the Identifier of your Segue in your Storyboard. You can set it by clicking on your Segue:
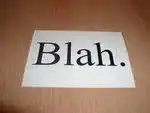
Keep in my mind that your ViewController needs to inherit from one more class:
class ViewController: UIViewController, UIGestureRecognizerDelegate {...}