Strings in PHP have an internal variable that saves the length of the string, so runtime of strlen($str)
is not depends on the length of the string at all.
Your problem is that you want to use mb_strlen
in order to get the number of characters in the string (and not the number of bytes). In other words - you want to know the length of the string, even if the string contains Unicode characters.
If you know that your string is UTF-8, it can be used for optimization. UTF-8 will save at most 4-bytes per char, so if you use isset($str[80])
- you know for sure that your string is at-least 20 chars (and probably much more). If not, you will still have to use the mb_
functions to get the information you need.
The reason for the usage of isset
instead of strlen
is because you asked about the optimized way. You can read more in this question regarding the two.
To sum it up - your optimized code would probably be:
if (isset($str[80]) || mb_strlen(mb_substr($str, 0, 21, 'utf-8'), 'utf-8') > 20) {
....
}
In php, the code will first check the isset
part, and if it return true the other part will not run (so you get the optimization here from both the isset
and the fact that you don't need to run the mb_
functions).
If you have more information about the characters in your string you can use it for more optimization (if, for example, you know that your all of the chars in your string are from the lower range of the UTF-8
, you don't have to use $str[80]
, you might as-well use $str[40]
.
You can use this table from wikipedia:

Together with the information from the utf8-chartable website:
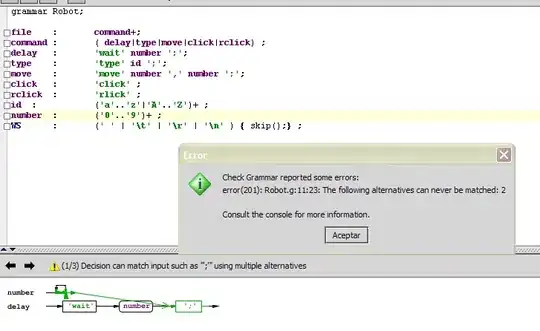
In order to help optimize the number of bytes you might need for each char in your string.