You could use the following little script, which uses OSX's built-in PHP which includes GD by default, so you would be staying native and not need ImageMagick or anything extra installed:
#!/usr/bin/php -f
<?php
// Get start image with transparency
$src = imagecreatefrompng('start.png');
// Get width and height
$w = imagesx($src);
$h = imagesy($src);
// Make a blue canvas, same size, to overlay onto
$result = imagecreatetruecolor($w,$h);
$blue = imagecolorallocate($result,0,0,255);
imagefill($result,0,0,$blue);
// Overlay start image ontop of canvas
imagecopyresampled($result,$src,0,0,0,0,$w,$h,$w,$h);
// Save result
imagepng($result,'result.png',0);
?>
So, if I start with this, which is transparent in the middle:
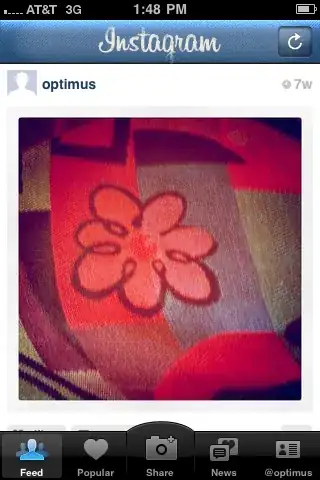
I get this as a result:
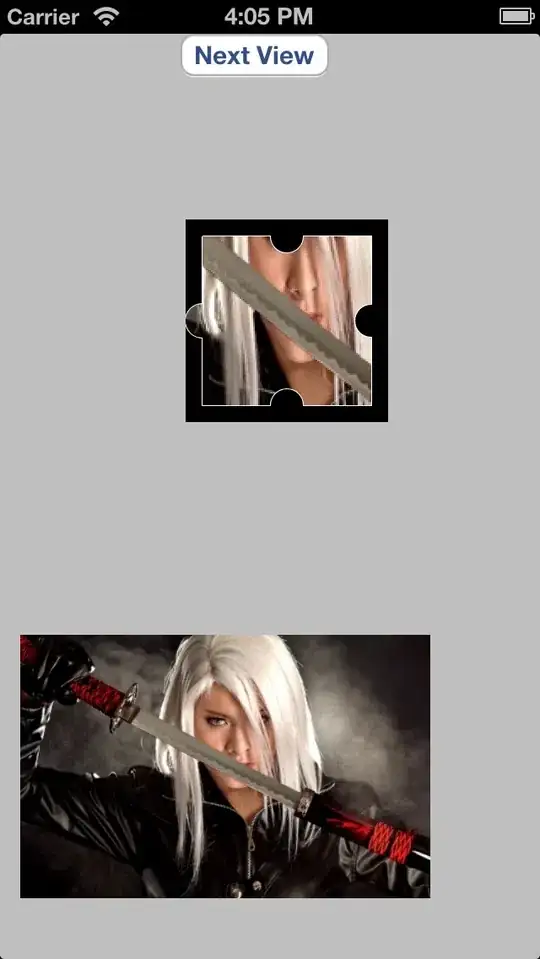
I made the canvas background blue so you can see it on StackOverflow's white background, but just change lines 12 & 13 for a white background like this:
...
...
// Make a white canvas, same size, to overlay onto
$result = imagecreatetruecolor($w,$h);
$white = imagecolorallocate($result,255,255,255);
imagefill($result,0,0,$white);
...
...
I did another answer here in the same vein to overcome another missing feature in sips
.