You can get a little more complex and use cowplot
to merge the plots together. You could automate this using lapply
to loop through your unique values, though that is probably overkill for just two groups.
library(ggplot2)
library(cowplot)
library(dplyr)
# Return to default theme, as cowplot sets its own
theme_set(theme_gray())
# Save y limits to get same scale
myYlims <- c(0, ceiling(max(df$sum)/100)*100)
# Generate each plot
x10 <-
ggplot(df %>%
filter(X == 10)
, aes(x = month, y = sum)) + geom_bar(stat = "identity") +
facet_grid(~ year,
switch = "x") +
panel_border() +
coord_cartesian(ylim = myYlims) +
xlab("X = 10")
x11 <-
ggplot(df %>%
filter(X == 11)
, aes(x = month, y = sum)) + geom_bar(stat = "identity") +
facet_grid(~ year,
switch = "x") +
panel_border() +
coord_cartesian(ylim = myYlims) +
xlab("X = 11")
# Put the plots together
plot_grid(x10
, x11 +
theme(axis.title.y = element_blank()
, axis.text.y = element_blank()
, axis.ticks.y = element_blank())
, rel_widths = c(1.1,1)
)

Here is an approach to automate this, including more complex data to justify the automation. Note that you will need to play with the aspect ratio of your output and with the rel_widths
option to make it look decent:
df <-
data.frame(
X = rep(1:6, each = 9)
, year = rep(rep(2016:2018, each = 3),3)
, month = rep(1:3, 6)
, sum = rnorm(9*6, 700, 100)
)
# Notice the variable type for month and year
df$month <- as.factor(df$month)
df$year <- as.factor(df$year)
df$X <- as.factor(df$X)
# Save y limits to get same scale
myYlims <- c(0, ceiling(max(df$sum)/100)*100)
# Generate each plot
eachPlot <- lapply(levels(df$X), function(thisX){
ggplot(df %>%
filter(X == thisX)
, aes(x = month, y = sum)) +
geom_bar(stat = "identity") +
facet_grid(~ year,
switch = "x") +
panel_border() +
coord_cartesian(ylim = myYlims) +
xlab(paste("X =", thisX))
})
# Remove axes from all but the first
eachPlot[-1] <- lapply(eachPlot[-1], function(x){
x +
theme(axis.title.y = element_blank()
, axis.text.y = element_blank()
, axis.ticks.y = element_blank()
)
})
# Put the plots together
plot_grid(plotlist = eachPlot
, rel_widths = c(1.4, rep(1, length(eachPlot)-1))
, nrow = 1
)
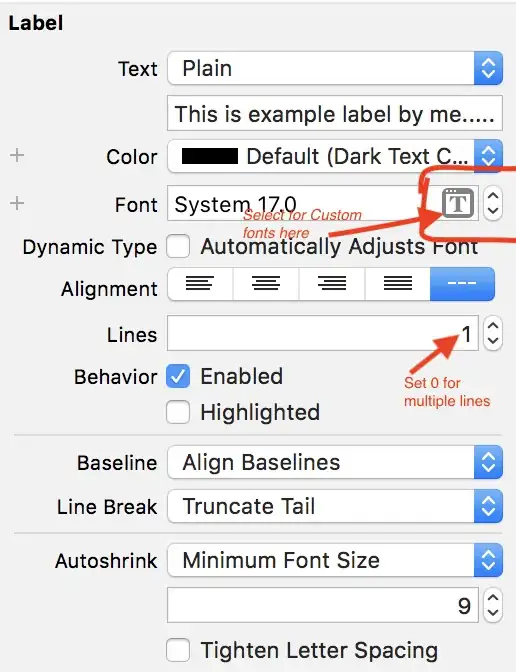