You can achieve what you want with one Regex.Replace
operation, but with a custom match evaluator:
var input = "4245 4 0 242 4424.09 0 422404 5955 0";
var results = Regex.Replace(input, @"(?:\s+|^)0(\s+)|(\s+0)$|\s+", m =>
m.Groups[1].Success ? ", " :
m.Groups[2].Success ? "" : ",");
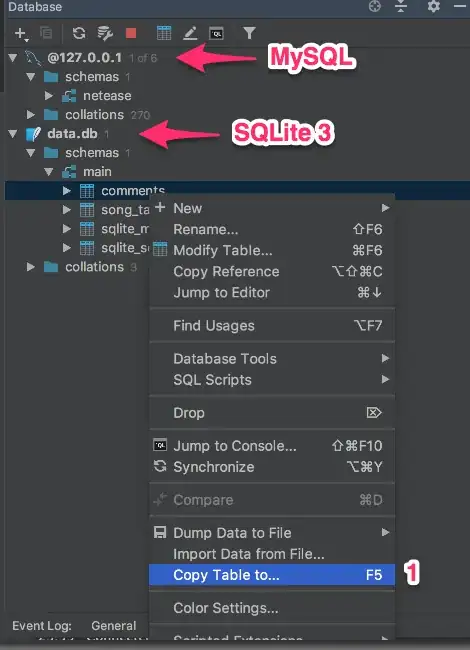
The point is to match those parts we need and capture into groups, so that they can be further analyzed and an appropriate action could be taken.
Pattern details:
(?:\s+|^)0(\s+)
- match 0
that is either at the start or with whitespaces before it and that is followed with 1 or more whitespaces (the whitespaces after 0
are stored in Group 1)
|
- or
(\s+0)$
- Group 2 capturing one or more whitespaces, then a 0
at the end ($
) of the string
|
- or
\s+
- (3rd option) 1 or more whitespaces in all other contexts.
And just in case one likes a more readable version, here is an alternative where the final 0
is removed with string methods, and then 1 regex is used to replace all spaces inside digits with a comma, but before we replace all 0
s with a mere String.Replace
.
var inp = "4245 4 0 0242 4424.09 0 422404 5955 0";
inp = inp.EndsWith(" 0") ? inp.Substring(0, inp.Length - 2) : inp;
var output = Regex.Replace(inp.Replace(" 0 ", ", "), @"(\d) (\d)", "$1,$2");