First things first - for-each
is nothing but syntactic sugar for Iterator
. Read this section of JLS. So, I will address this question as a simple FOR loop vs Iterator.
Now, when you use Iterator
to traverse over a collection, at bare minimum you will be using two method - next()
and hasNext()
, and below are their ArrayList
implementations:
public boolean hasNext() {
return cursor != size;
}
@SuppressWarnings("unchecked")
public E next() {
checkForComodification();
int i = cursor;
if (i >= size)
throw new NoSuchElementException();
Object[] elementData = ArrayList.this.elementData;
if (i >= elementData.length)
throw new ConcurrentModificationException();
cursor = i + 1;
return (E) elementData[lastRet = I]; // hagrawal: this is what simple FOR loop does
}
Now, we all know the basic computing that there will be performance difference if on the processor I have to just execute myArray[i]
v/s complete implementation of next()
method. So, there has to be a difference in performance.
It is likely that some folk might come back strongly on this, citing performance benchmarks and excerpts from Effective Java, but the only other way I can try to explain is that this is even written in Oracle's official documentation - please read below from RandomAccess
interface docs over here.
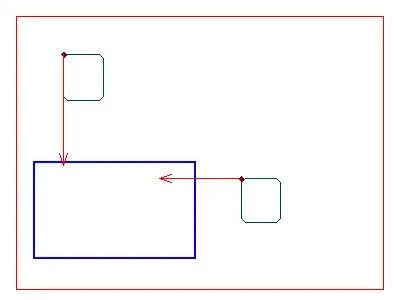
It is very clearly mentioned that there will be differences. So, if you can convince me that what is written in official docs is wrong and will be changed, I will be ready to accept the argument that there is no performance difference between simple FOR loop and Iterator or for-each.
So IMHO, correct way to put this whole argument is this:
- If the collection implements
RandomAccess
interface then simple FOR loop will perform (at least theoretically) better than Iterator or for-each. (this is what is also written in RandomAccess docs)
- If the collection doesn't implement
RandomAccess
interface then Iterator or for-each will perform (for sure) better than simple FOR loop.
- However, for all practical purposes, for-each is the best choice in general.