Using some example data to generate a plot that (I think) shows the problem you're having.
library(ggplot2)
df <- data.frame(val = c(10, 20, 100, 5), name = LETTERS[1:4])
ggplot(df, aes(x = name, y = val, fill = name)) +
geom_bar(stat = "identity")
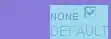
There is a gap from the zero point on the y axis (bottom of the bars) and where the x axis labels are.
You can remove this using scale_y_discrete
or scale_y_continuous
, depending on the nature of your data, and setting expand
to c(0,0)
:
ggplot(df, aes(x = name, y = val, fill = name)) +
geom_bar(stat = "identity") +
scale_y_discrete(expand = c(0,0)) +
scale_x_discrete(expand = c(0,0))
This gives the plot:
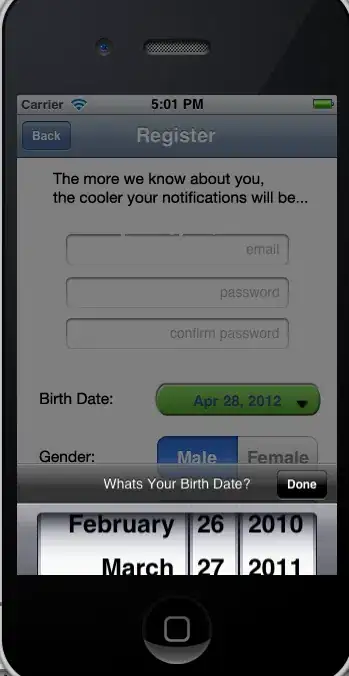
Note I've also removed the gap along the y axis, simply remove the scale_x_discrete
line to add this gap back in.
Since error bars are an issue, here are a few examples:
ggplot(df, aes(x = name, y = val, fill = name)) +
geom_bar(stat = "identity") +
geom_errorbar(aes(ymin = val - 10,
ymax = val + 10))

You can use scale to remove the padding down to the error bar:
ggplot(df, aes(x = name, y = val, fill = name)) +
geom_bar(stat = "identity") +
geom_errorbar(aes(ymin = val - 10,
ymax = val + 10)) +
scale_y_continuous(expand = c(0,0))
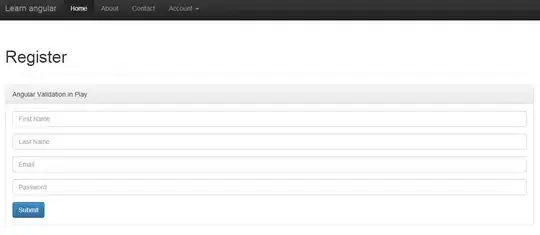
Or you can use coord_cartesian to give a hard cutoff:
ggplot(df, aes(x = name, y = val, fill = name)) +
geom_bar(stat = "identity") +
geom_errorbar(aes(ymin = val - 10,
ymax = val + 10)) +
scale_y_continuous(expand = c(0,0)) +
coord_cartesian(ylim = c(0, max(df$val) + 10))
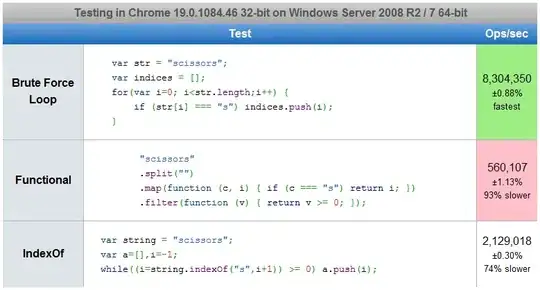