Here's a ggplot2
approach that comes close to the plot format in your question:
library(ggplot2)
# Fake data
set.seed(1234)
nobs<-52
t<-seq(1,nobs)
x<-rnorm(nobs,0,10) + t + 20
dat = data.frame(year=rep(2004:2016, each=4), qtr=rep(1:4, 13), x = x)
ggplot(dat, aes(paste(year,qtr), x)) +
geom_vline(xintercept=seq(4.5,100,4), colour="grey80",lwd=0.3) +
geom_line(aes(group=1)) +
annotate(seq(2.5, 2.5 + 12*4, length.out=13), -2, label=2004:2016,
geom="text", colour="grey30", size=3.5) +
theme_bw(base_size=10) +
theme(axis.text.x=element_text(angl=-90, vjust=0.5, colour="grey30"),
panel.grid.major.x=element_blank()) +
scale_x_discrete(name="Quarter",
labels=rep(paste0(month.abb[seq(1,12,3)],"-",month.abb[seq(3,12,3)]),20)) +
coord_cartesian(xlim=c(0,13*4 + 1), ylim=c(-4,max(dat$x + 2)), expand=FALSE)
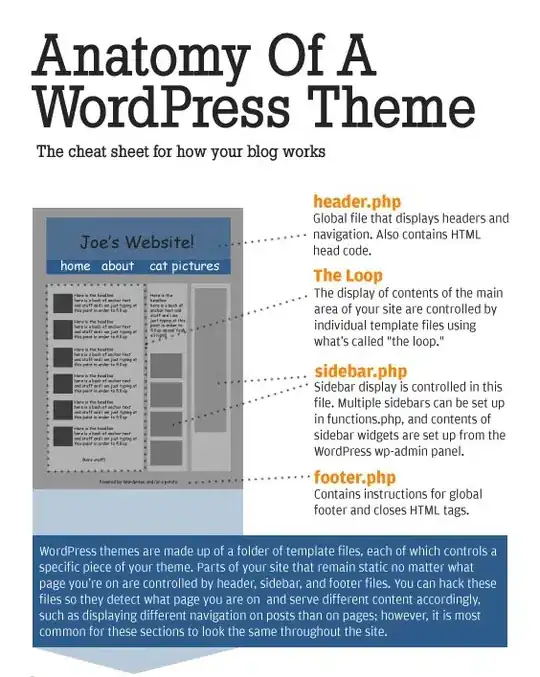
Facetting comes closer to the formatting you wanted, but there's no way (AFAIK) within the normal ggplot workflow to connect lines across the facets. In the plot below, the vertical lines show the borders between each facet. There are (somewhat complicated) ways to try and connect lines across facets, but I didn't make it that far in the example below.
dat$qtr = rep(paste0(month.abb[seq(1,12,3)],"-",month.abb[seq(3,12,3)]),13)
dat$qtr = factor(dat$qtr, levels=paste0(month.abb[seq(1,12,3)],"-",month.abb[seq(3,12,3)]))
p=ggplot(dat, aes(qtr, x)) +
geom_line(aes(group=1)) +
coord_cartesian(xlim=c(0.5,4.5), expand=FALSE, ylim=c(-2,80)) +
facet_grid(. ~ year, switch="x") +
annotate(x=c(0.5, 4.5), xend=c(0.5,4.5), y=-16, yend=-2, geom="segment",
colour="grey70", size=0.3) +
theme_bw(base_size=10) +
theme(panel.margin=unit(0,"lines"),
axis.text.x=element_text(angl=-90, vjust=0.5, colour="grey30"),
panel.border=element_rect(colour="grey70", size=0.3),
panel.grid.major=element_blank(),
strip.background=element_rect(fill="grey90", colour="grey20"),
axis.line=element_line(colour="black")) +
labs(x="Quarter")
# Turn off clipping and draw plot
gt <- ggplot_gtable(ggplot_build(p))
gt$layout$clip[gt$layout$name=="panel"] <- "off"
grid.draw(gt)
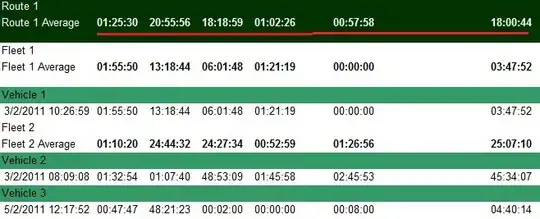