Heads up!
You need to add a name
attribute to all your <input>
fields as in:
<input type="text" id="item1Name" value="Item 1" class="itemNames" name="itemNames">
Also, use <form>
instead of <fieldset>
unless you do have explicit reason for such a purpose.
The syntax for serializeArray();
is as follow: $(selector).serializeArray();
.
Reference: w3schools (read on Definition and Usage ).
Same concept used here with the intention to serialize a collection of alike item.
Please note: using of the <form>
element is not compulsory but simply recommended to get you easily and effectively serialize based on your intended purpose and information shared above in your post; I might have misread you on that, but the most important thing missing out was the name
attribute, which accounts for your empty array []
as there's nothing available to be serialized.
Remember that based on your project requirements, you may have to make use of <fieldset>
as and when required.
You may use the <fieldset>
element with different ids to group related items you want to identify as a unit. As such, you may have something like this:
<form id="itemDetails">
<fieldset id="itemInformation">
<!-- itemInformation label and input fields -->
</fieldset>
<fieldset id="itemOrigin">
<!-- itemOrigin label and input fields -->
</fieldset>
<fieldset id="itemDestination">
<!-- itemDestination label and input fields -->
</fieldset>
</form>
You can then use $('#itemDetails').serializeArray();
to serialize your entire form or $('#FIELDSET_ID').serializeArray();
where FIELDSET_ID is the corresponding id of a selected fieldset you want to serialize out of your form based on events so as to achieve your intended purpose.
Added the snippet below to illustrate how you can effectively pair up an item with it's corresponding value;
$('#itemInformation .nextButton').bind('click', function (event) {
event.preventDefault();
console.table($('#itemInformation').serializeArray());
console.log($('#itemInformation').serializeArray());
});
<form id="itemDetails">
<!-- grouping all fieldset under a form element with an assigned id where
they can all be hierarchically referenced for precision where needed -->
<fieldset id="itemInformation">
<h2>Items</h2>
<div class="itemGroup">
<input type="number" id="item1Name" placeholder="0.00" class="itemNames" name="item1Name">
</div>
<div class="itemGroup">
<input type="number" id="item2Name" placeholder="0.00" class="itemNames" name="item2Name">
</div>
<div class="itemGroup">
<input type="number" id="item3Name" placeholder="0.00" class="itemNames" name="item3Name">
</div>
<div class="changeNumber">
<button class="increase">+</button>
<button class="decrease">–</button>
</div>
<div class="buttons">
<button class="previousButton btn">Previous</button>
<button class="nextButton btn">Next</button>
</div>
</fieldset>
<!-- other fieldset as necessary and harvest their input separately in
a similar fashion as done with the first one itemInformation -->
<fieldset id="itemOrigin">
<!-- itemOrigin label and input fields -->
</fieldset>
<fieldset id="itemDestination">
<!-- itemDestination label and input fields -->
</fieldset>
</form>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script>
Below, a sample output of the above in a browser developer console (here: Google Chrome Browser);
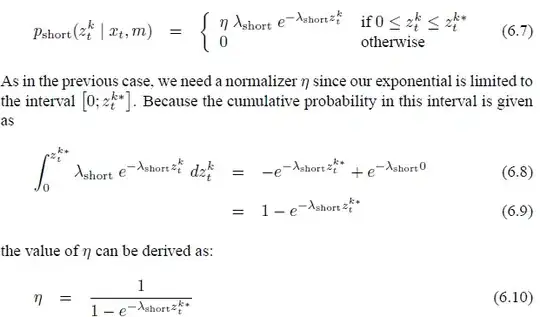
Also, available here (JSfiddle).
You will realise that each <div class="itemGroup">
has a single <input>
element which has no value
attribute but a placeholder
to illustrate the expected input format. You may use value
instead in case you want to pre-define a fixed value to be paired up against a corresponding item should there be no value entered. The name
attribute is necessary: do not omit it.
As such (as above), when you serialize, the values collected from your input field will be paired up with the corresponding entered value and or default one(s).