Try my answer its work perfectly.
var record : NSArray = NSArray()
var hight: CGFloat = 0.0
Put this code in viewDidLoad()
record = ["I have a UITextView in a custom UITableViewCell. The textview delegate is assigned in the tableviewcell custom class." ,"Textview scrolling is disabled. Text loads into each textview and is multiline. But the text is always clipped because the cell height doesn't change.","I have the following in viewDidLoad of the tableview controller:"," have a UITextView in a custom UITableViewCell. The textview delegate is assigned in the tableviewcell custom class.","Textview scrolling is disabled. Text loads into each textview and is multiline. But the text is always clipped because the cell height doesn't change.","I have the following in viewDidLoad of the tableview controller:","i just give you one link at put place i use label and you can now use your textview and give same constrain that i give in that link and try it so your problem will be solve","I have the following in viewDidLoad of the tableview controller:"];
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return record.count
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("Textviewcell", forIndexPath: indexPath)
let textview: UITextView = (cell.viewWithTag(5) as! UITextView)
textview.text = record.objectAtIndex(indexPath.row) as? String
return cell
}
func tableView(tableView: UITableView, heightForRowAtIndexPath indexPath: NSIndexPath) -> CGFloat {
// 7.1>
hight = self.findHeightForText(self.record.objectAtIndex(indexPath.row) as! String, havingWidth: self.view.frame.size.width - 10, andFont: UIFont.systemFontOfSize(14.0)).height
return 44 + hight
}
func findHeightForText(text: String, havingWidth widthValue: CGFloat, andFont font: UIFont) -> CGSize {
var size = CGSizeZero
if text.isEmpty == false {
let frame = text.boundingRectWithSize(CGSizeMake(widthValue, CGFloat.max), options: .UsesLineFragmentOrigin, attributes: [NSFontAttributeName: font], context: nil)
size = CGSizeMake(frame.size.width, ceil(frame.size.height))
}
return size
}
In Swift 3.0
func findHeightForText(text: String, havingWidth widthValue: CGFloat, andFont font: UIFont) -> CGSize {
var size = CGSize.zero
if text.isEmpty == false {
let frame = text.boundingRect(with: CGSize(width: widthValue, height: CGFloat.greatestFiniteMagnitude), options: .usesLineFragmentOrigin, attributes: [NSFontAttributeName: font], context: nil)
size = CGSize(width: frame.size.width, height: ceil(frame.size.height))//CGSizeMake(frame.size.width, ceil(frame.size.height))
}
return size
}
Here are some screen shot .Storyboard
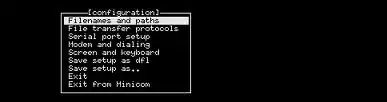
Runtime tableview with UITextView
