Not sure I completely understood your question but it sounds like you want to build off the inputs for sand, silt and clay % and the subsequent soil type determination within your macro.
I changed the formatting of your input cells from 'Percentage' to 'General' and input percentages so that 20 is 20%, 15 is 15%, etc. The inequalities on Sheet ("Calculations") for basic categorization of soil following the soil texture triangle were hard coded in an if/elseif statement. See if this helps:
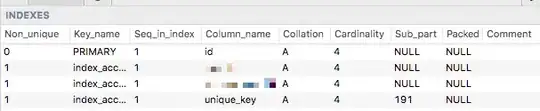
Sub Test()
Dim i As Integer: Dim sand_percent As Long: Dim clay_percent As Long:
Dim silt_percent As Long:
Dim usda_texture As Variant:
For i = 4 To 7
With Sheets("Texture Calculator")
sand_percent = Range("B" & i).Value
clay_percent = Range("D" & i).Value
silt_percent = Range("F" & i).Value
usda_texture = Texture_Determination(sand_percent, clay_percent, silt_percent)
Range("G" & i).Value = usda_texture
End With
Next i
End Sub
Function Texture_Determination(Sand As Long, Clay As Long, Silt As Long) As Variant
If Sand + Clay + Silt <> 100 Then
MsgBox "Please ensure your inputs add up to 100%"
Exit Function
End If
'Determine the kind of soil texture using if/elseifs
If (Silt + 1.5 * Clay) < 15 Then
soil = "Sand"
ElseIf Silt + 1.5 * Clay >= 15 And (Silt + 2 * Clay < 30) Then
soil = "Loamy Sand"
ElseIf ((Clay >= 7 And Clay < 20) And (Sand > 52) And ((Silt + 2 * Clay) >= 30) Or (Clay < 7 And Silt < 50 And (Silt + 2 * Clay) >= 30)) Then
soil = "Sandy Loam"
ElseIf ((Clay >= 7 And Clay < 27) And (Silt >= 28 And Silt < 50) And (Sand <= 52)) Then
soil = "Loam"
ElseIf ((Silt >= 50 And (Clay >= 12 And Clay < 27)) Or ((Silt >= 50 And Silt < 80) And Clay < 12)) Then
soil = "Silt"
ElseIf ((Clay >= 20 And Clay < 35) And (Silt < 28) And (Sand > 45)) Then
soil = "Sandy Clay Loam"
ElseIf ((Clay >= 27 And Clay < 40) And (Sand > 20 And Sand <= 45)) Then
soil = "Clay Loam"
ElseIf ((Clay >= 27 And Clay < 40) And (Sand <= 20)) Then
soil = "Silty Clay Loam"
ElseIf (Clay >= 35 And Sand > 45) Then
soil = "Sandy Clay"
ElseIf (Clay >= 40 And Silt >= 40) Then
soil = "Silty Clay"
ElseIf (Clay >= 40 And Sand <= 45 And Silt < 40) Then
soil = "Clay"
Else
soil = "Could not determine soil texture"
End If
Texture_Determination = soil
End Function