JavaFx is mostly composed of set of [well designed] tools but unfortunately by itself does not provide a good framework for creating complex UI designs e.g. MVC/MVP patterns, view flows and actions on multiple controllers, So you have to rely on third-party application frameworks for those for example:
In my opinion none of them are widely used or mature enough to be considered a de facto standard but using them is encouraged.
Example Using DataFx
DataFx uses a concept named Flow to associate views sharing a flow of events (and possibly data) among themselves. By using Flow combined with EventSystem you can define and access methods on other controllers and assign custom event listeners to various events associated with JavaFx Nodes in different controllers sharing a flow.
Here is an example from DataFx samples which represents two separate sender and receiver views with distinct FXML files and controllers:
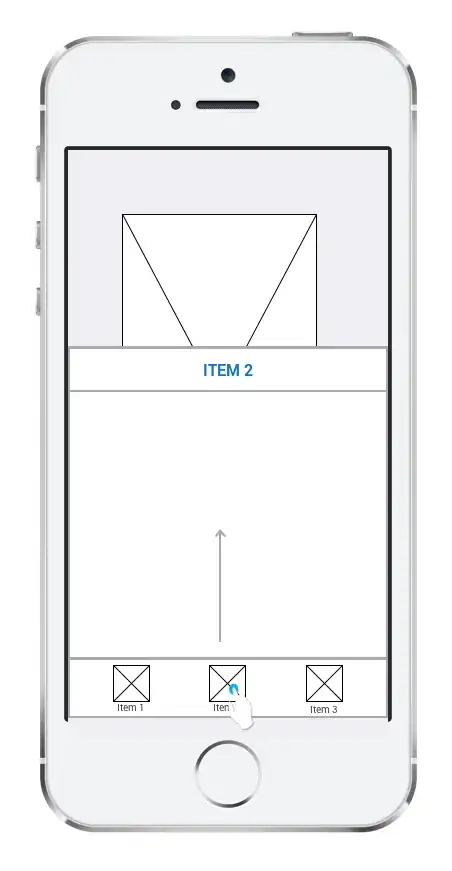
public class EventSystemDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
HBox box = new HBox();
box.setSpacing(12);
box.setPadding(new Insets(12));
box.setFillHeight(true);
box.setAlignment(Pos.CENTER);
Flow senderFlow = new Flow(ProducerController.class);
box.getChildren().add(senderFlow.start());
Flow receiverFlow = new Flow(ReceiverController.class);
box.getChildren().add(receiverFlow.start());
primaryStage.setScene(new Scene(box));
primaryStage.show();
}
public static void main(String... args) {
launch(args);
}
}
Sender view controller:
@ViewController("producer.fxml")
public class ProducerController {
@FXML
@EventTrigger()
private Button sendButton;
@FXML
private TextField textField;
@EventProducer("test-message")
private String getMessage() {
return textField.getText();
}
}
Receiver view controller:
@ViewController("receiver.fxml")
public class ReceiverController {
@FXML
private Label messageLabel;
@OnEvent("test-message")
private void onNewChatMessage(Event<String> e) {
messageLabel.setText(e.getContent());
}
}
Sender View:
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.text.*?>
<?import javafx.geometry.*?>
<?import javafx.scene.control.*?>
<?import java.lang.*?>
<?import javafx.scene.layout.*?>
<VBox alignment="TOP_CENTER" maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" spacing="12.0" style="-fx-border-color: darkgrey; -fx-border-width: 2;" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1">
<children>
<Label text="Sender">
<font>
<Font name="System Bold" size="24.0" />
</font>
</Label>
<TextField fx:id="textField" />
<Button fx:id="sendButton" mnemonicParsing="false" text="send" />
</children>
<padding>
<Insets bottom="12.0" left="12.0" right="12.0" top="12.0" />
</padding>
</VBox>
Receiver View:
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.text.*?>
<?import javafx.geometry.*?>
<?import javafx.scene.control.*?>
<?import java.lang.*?>
<?import javafx.scene.layout.*?>
<VBox alignment="TOP_CENTER" maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" spacing="12.0" style="-fx-border-color: darkgrey; -fx-border-width: 2;" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1">
<children>
<Label text="Receiver">
<font>
<Font name="System Bold" size="24.0" />
</font></Label>
<Label fx:id="messageLabel" />
</children>
<padding>
<Insets bottom="12.0" left="12.0" right="12.0" top="12.0" />
</padding>
</VBox>
For further reading:
http://www.oracle.com/technetwork/java/javafx/community/3rd-party-1844355.html
http://www.guigarage.com/2015/02/quick-overview-datafx-mvc-flow-api/
http://www.guigarage.com/2014/05/datafx-8-0-tutorials/
http://jacpfx.org/2014/11/03/JacpFX_DataFX_a_perfect_match.html
Passing Parameters JavaFX FXML