You can apply some constraint on the minAreaRect
containing the edge.
You can find an example here, but since your edges touch the border, you need an additional trick to make findContours
work correctly, so below the improved code.
With a simple constraint on the aspect ratio, you get:
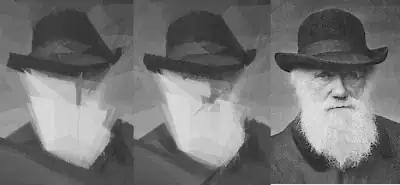
where you removed the red edges:
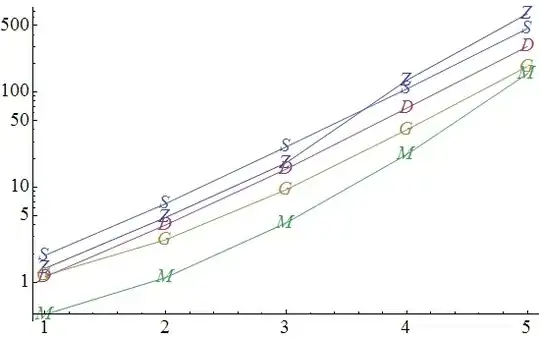
You can add additional contraint, e.g. on the height
, to fit your specific purposes.
Here the code:
#include<opencv2/opencv.hpp>
using namespace cv;
int main()
{
// Load image
Mat1b img = imread("path_to_image", IMREAD_GRAYSCALE);
// Remove JPG artifacts
img = img > 200;
Mat1b result = img.clone();
// Create output image
Mat3b out;
cvtColor(img, out, COLOR_GRAY2BGR);
// Find contours
Mat1b padded;
copyMakeBorder(img, padded, 1, 1, 1, 1, BORDER_CONSTANT, Scalar(0));
vector<vector<Point>> contours;
findContours(padded, contours, RETR_LIST, CHAIN_APPROX_NONE, Point(-1, -1));
for (const auto& contour : contours)
{
// Find minimum area rectangle
RotatedRect rr = minAreaRect(contour);
// Compute aspect ratio
float aspect_ratio = min(rr.size.width, rr.size.height) / max(rr.size.width, rr.size.height);
// Define a threshold on the aspect ratio in [0, 1]
float thresh_ar = 0.05f;
// Define other constraints
bool remove = false;
if (aspect_ratio < thresh_ar) {
remove = true;
}
// if(some_other_constraint) { remove = true; }
Vec3b color;
if (remove) {
// Almost straight line
color = Vec3b(0, 0, 255); // RED
// Delete edge
for (const auto& pt : contour) {
result(pt) = uchar(0);
}
}
else {
// Curved line
color = Vec3b(0, 255, 0); // GREEN
}
// Color output image
for (const auto& pt : contour) {
out(pt) = color;
}
}
imshow("Out", out);
imshow("Result", result);
waitKey();
return 0;
}