This is a very good question.
To really understand this, you better know how C codes are executed in computer:
First, the compiler will compile the C code into assembly code, then assembly codes will be translated into machine code, which can run in main memory directly.
As for your code:
void main() {
int i, j=6;
for(; i=j ; j-=2 )
printf("%d",j);
}
To figure out why the result is 642, We want to see its assembly code.
Using VS debugging mode, we can see:
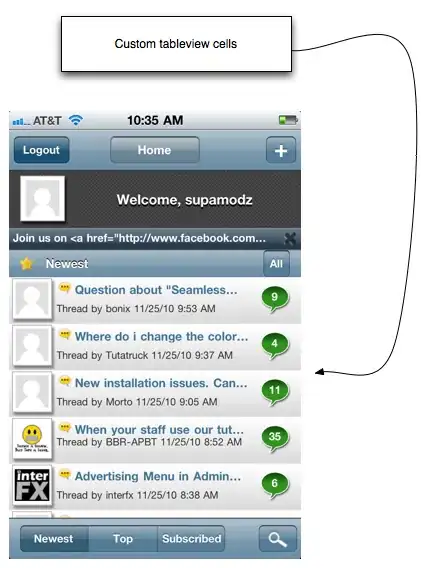
Especially look at this:
010217D0 mov eax,dword ptr [j]
010217D3 mov dword ptr [i],eax
010217D6 cmp dword ptr [i],0
010217DA je main+4Fh (010217EFh)
The four lines of assembly code correponding to the C code "i=j", it means, first move the value of j to register eax, then move the value of register eax to i(since computer can not directly move the value of j to i, it just use register eax as a bridge), then compare the value of i with 0, if they are equal, jump to 010217EFh, the loop ends; if not, the loop continues.
So actually it's first an assignment, then a comparision to decide whether the loop is over; as 6 declines to 0 ,the loop finally stops, I hope this can help you understand why the result is 642 :D