Put together this hackety-hack-hack to make this work (even works with xdebug remote debugging if you set it up!!!).
UPDATE: one caveat with this solution is when you terminate the running CLI in Eclipse, it's terminating the wrapper script, not the php server directly. I've added some trapping and forawding of signals to child (php server) process. Works in OSX.
Overview:
- I'm running Eclipse Neon
- Need a router file in document root you wish to serve from (see this: http://php.net/manual/en/features.commandline.webserver.php)
- Create a wrapper bash script to call PHP in server mode and pass in details
- Set script to have executable permissions
- Add this bash script as a PHP executable
- For the project, create a run configuration as PHP CLI, using this new executable, passing the router file in.
Here's the bash script php5.6-server
:
#!/bin/bash
_sigterm() {
echo "Caught SIGTERM signal!"
kill -2 "$child"
}
_sigint() {
echo "Caught SIGINT signal!"
kill -14 "$child"
}
if [ $1 = "-v" ]; then
#This is needed for when eclipse trys to detect php version
/path/to/php -v
else
trap _sigterm SIGTERM
trap _sigint SIGINT
# This is why your router file needs to be in the doc root
ROUTER=${@: -1}
DIR=$(dirname $ROUTER)
/path/to/php -S localhost:8000 -t $DIR $ROUTER
child=$!
wait "$child"
fi
Here's a simple router.php
just to get it working:
<?php
// router.php
if (preg_match('/\.(?:png|jpg|jpeg|gif)$/', $_SERVER["REQUEST_URI"])) {
return false; // serve the requested resource as-is.
} else {
echo "<p>Welcome to PHP</p>";
}
Now in Eclipse go to Eclipse->Preferences->PHP->PHP Executables
and add a new server:
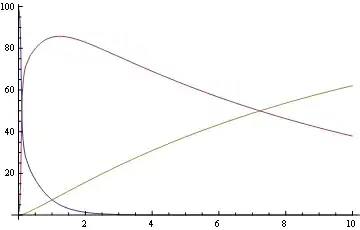
And that should be it. Now create a PHP CLI Run configurations using the wrapper executable as 'Alternate PHP' and for the php file specify the route file:
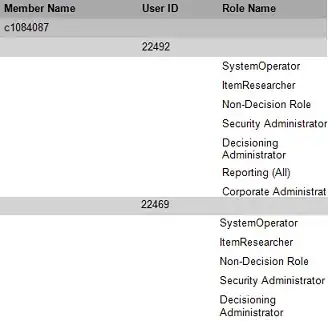
Then Run as CLI!!! A PHP server should now be listening on port 8000 on your localhost. I suspect this method may also work for HHVM's Proxygen server.