this can be done by manipulating the prettify
function of ionRangeSlider, which returns the slider labels for each value in the range of selectable slider values. Simply create a file slider.js
as following (enter your slider ID and lowest selectable value at marked spots) and include it in your app with includeScript('slider.js')
:
$(document).ready(function() {
/**
Custom slider labels
**/
// Convert numbers of min to max to "lower" and "higher"
function returnLabels(value) {
// remove label of selected
$('.my_slider').find('.irs-single').remove();
// $('.my_slider').find('.irs-grid-text').remove(); // this is an alternative to ticks=F
if (value === 0){ // enter your lowest slider value here
return "lower";
}else{
return "higher";
}
}
var someID = $("#YOUR_SLIDER_ID").ionRangeSlider({ // enter your shiny slider ID here
prettify: returnLabels,
force_edges: true,
grid: false
});
});
Then, wrap your slider in a div with class "my_slider":
library(shiny)
ui <- fluidPage(
includeScript("slider.js"),
div(class="my_slider", # to be able to manipulate it with JQuery
sliderInput("YOUR_SLIDER_ID",
"Slider Value:", ticks = F,
min = 0, max = 50, value = 30))
)
server <- function(input, output) {}
shinyApp(ui, server)
The result looks as follows:
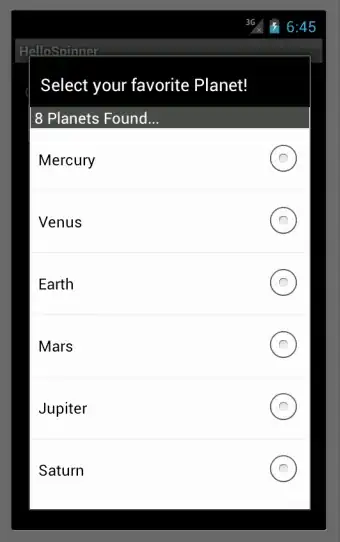
To fix the bug that labels disappear when min/max value is selected, define this UIoutput in your app:
# Just a small fix to reactivate Labels when Min/Max value is chosen
output$fixSlider <- renderUI({
# declare dependency on slider
input$YOUR_SLIDER_ID
minVis <- "$('.my_slider').find('.irs-min').attr('style','visibility: visible');"
maxVis <- "$('.my_slider').find('.irs-max').attr('style','visibility: visible');"
return(tags$script(paste(minVis,maxVis)))
})
You're welcome :-)