A DataTemplateSelector
can do what I think you want. You can define different templates from your user control's XAML, then have the selector choose among them.
Assume these model classes:
public class Employee
{
public Employee(string fullName)
{
FullName = fullName;
}
public string FullName { get; }
}
public class Certifying
{
public Certifying(string certifyingAuthor, DateTime certifyingDate)
{
CertifyingAuthor = certifyingAuthor;
CertifyingDate = certifyingDate;
}
public string CertifyingAuthor { get; }
public DateTime CertifyingDate { get; }
}
Your user control's XAML has a ListBox
, which in turn uses a template selector (that we'll get to in a moment) -- and also defines different templates for the two different model types:
<UserControl
x:Class="WPF.UserControl1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WPF"
>
<Grid>
<ListBox x:Name="listBox">
<ListBox.ItemTemplateSelector>
<local:MyTemplateSelector>
<local:MyTemplateSelector.EmployeeTemplate>
<DataTemplate DataType="local:Employee">
<TextBlock
Foreground="Red"
Text="{Binding FullName}"
/>
</DataTemplate>
</local:MyTemplateSelector.EmployeeTemplate>
<local:MyTemplateSelector.CertifyingTemplate>
<DataTemplate DataType="local:Certifying">
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="120" />
<ColumnDefinition Width="Auto" />
</Grid.ColumnDefinitions>
<TextBlock
Foreground="Blue"
Text="{Binding CertifyingAuthor}"
/>
<TextBlock
Grid.Column="1"
Foreground="Green"
Text="{Binding CertifyingDate}"
/>
</Grid>
</DataTemplate>
</local:MyTemplateSelector.CertifyingTemplate>
</local:MyTemplateSelector>
</ListBox.ItemTemplateSelector>
</ListBox>
</Grid>
</UserControl>
The user control's code-behind, where I just assign a list of model objects to the list box (for simplicity):
public partial class UserControl1
{
public UserControl1()
{
InitializeComponent();
listBox.ItemsSource = new List<object>
{
new Employee("Donald Duck"),
new Certifying("Mickey Mouse", DateTime.Now),
new Employee("Napoleon Bonaparte"),
new Certifying("Homer Simpson", DateTime.Now - TimeSpan.FromDays(2)),
};
}
}
And finally, the template selector. Its two properties were set from the user control's XAML; the logic of SelectTemplate
decides which one to apply:
public class MyTemplateSelector : DataTemplateSelector
{
/// <summary>
/// This property is set from XAML.
/// </summary>
public DataTemplate EmployeeTemplate { get; set; }
/// <summary>
/// This property is set from XAML.
/// </summary>
public DataTemplate CertifyingTemplate { get; set; }
public override DataTemplate SelectTemplate(object item, DependencyObject container)
{
if (item is Employee)
{
return EmployeeTemplate;
}
if (item is Certifying)
{
return CertifyingTemplate;
}
return base.SelectTemplate(item, container);
}
}
And, for completeness, usage of the user control:
<Grid>
<wpf:UserControl1 />
</Grid>
The end result, where Napoleon is currently selected, and Homer suffers a mouse-over:
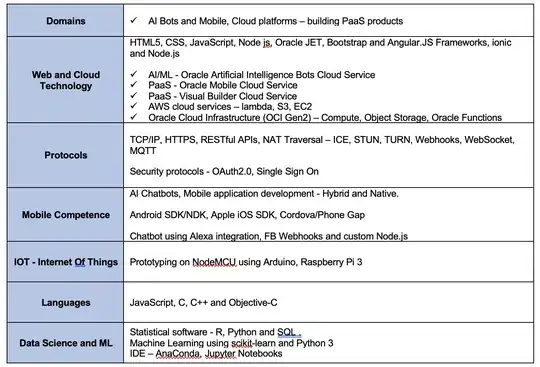