x
is a string with \r\n
line separators. Each "line" is not delimited in a standard way (tab, for instance) but rather by multiple spaces. There's probably an elegant way to handle this.
The first line is empty, the second line is headers, third line is just a row of -----
, so you need to process from line 4 to the end.
import subprocess
import re
p = subprocess.Popen('netsh interface show interface',stdout=subprocess.PIPE)
[x, err] = p.communicate()
items = x.split('\r\n')
for itm in items[3:]:
## convert this to a delimited line for ease of processing:
itm = re.sub('\s\s+', '\t', itm)
print(itm.split('\t')[-1])
Results:
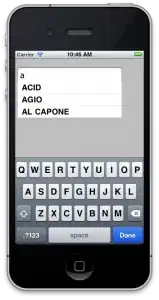
Or further refined:
import subprocess
import re
p = subprocess.Popen('netsh interface show interface',stdout=subprocess.PIPE)
[x, err] = p.communicate()
items = x.split('\r\n')
print('\n'.join(map(lambda itm: re.sub('\s\s+', '\t', itm).split('\t')[-1], items[3:])))
Update from Comments
so it works as intended although if I have like 4 Interfaces and I just want to read first one, what would be best way to do that? I tried looking into regex but couldn't find a way to just keep first line and remove rest from results.
You won't need regex for this. The only thing I used regex for was to convert any instance of multiple space character, to a \t
(tab) character, so that we can easily parse the lines using split
. Otherwise, as you've observed, it will produce outputs like 'Local', 'Area', 'Network'
So the approach I have above assumed you wanted to print out all of the names, and we determined that these items begin on line 4 of the stdout. That's why we did:
for itm in items[3:]:
That line says "iterate over each thing in items
, from the third thing until the very last thing." This is a concept known as slicing which can be done on any sequence object (string, tuple, list, etc.)
I have below for connections and just want to know first connection name and remove rest from my result.
So we no longer need iteration (which is done if we need to process each item). We know we only need one item, and it's the first item, so you can revise as:
import subprocess
import re
p = subprocess.Popen('netsh interface show interface',stdout=subprocess.PIPE)
[x, err] = p.communicate()
## This gets the line-delimited list from stdout
items = x.split('\r\n')
## Now, we only need the fourth item in that list
itm = items[3]
## convert this to a delimited line for ease of processing:
itm = re.sub('\s\s+', '\t', itm)
## print to console
print(itm.split('\t')[-1])
Or, more succinctly:
import subprocess
import re
p = subprocess.Popen('netsh interface show interface',stdout=subprocess.PIPE)
[x, err] = p.communicate()
## This gets the line-delimited list from stdout
items = x.split('\r\n')
"""
Now, we only need the fourth item in that list
convert this to a delimited line for ease of processing,
and finally print to console
This is a one-line statement that does what 3 lines did above
"""
print(re.sub('\s\s+', '\t', items[3]).split('\t')[-1])